Little Gyro has just found an empty integer set A in his right pocket, and an empty integer set B in his left pocket. At the same time, Little Gyro has already thought about two integers n, m in his mind. As Little Gyro is bored, he decides to play with these two sets. Then, Little Gyro is ready to divide the integer series 1,2,3...m−1,m to these two empty sets. With the following rules: If the number is an integer multiple of n, Little Gyro will put it in set A. Otherwise, Little Gyro will put it in set B instead. Now given two integers n, m, after these operations, Little Gyro wants to know the result of sum(B)−sum(A) and ask you for help, please help him to calculate. Input Specification: There are multiple test cases. The first line of the input contains an integer T (1 ≤ T ≤ 10 5 ), indicating the number of test cases. For each test case: Each line contains two integers n, m (1 ≤ n, m ≤ 10 9 ), indicating the length of the sequence. Output Specification: For each test case output an integer indicating the result of sum(B)−sum(A). Sample Input: 3 3 5 2 10 3 16 Sample Output: 9 -5 46 Hint: For the first sample, after these operations, A = <3>, B = <1,2,4,5>, and the answer is 9. For the second sample, after these operations, A = <2,4,6,8,10>, B = <1,3,5,7,9>, and the answer is -5.
时间: 2024-02-01 17:03:35 浏览: 223
To solve this problem, we can first calculate the sum of integers in set A and the sum of integers in set B separately.
The sum of integers in set A is the sum of all integer multiples of n within the range of 1 to m. We can calculate this by first finding the number of integer multiples of n within the range of 1 to m (which is floor(m/n)), and then multiplying it by n.
The sum of integers in set B is the sum of all integers within the range of 1 to m, minus the sum of integers in set A. We can calculate this by using the formula for the sum of an arithmetic series.
Finally, we can return the difference between the sum of integers in set B and the sum of integers in set A as the answer.
Here's the Python code to implement this algorithm:
```python
t = int(input())
for i in range(t):
n, m = map(int, input().split())
a_sum = (m // n) * n * (n + 1) // 2
b_sum = m * (m + 1) // 2 - a_sum
print(b_sum - a_sum)
```
The time complexity of this algorithm is O(T), where T is the number of test cases.
阅读全文
相关推荐
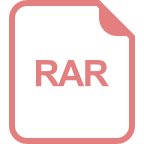
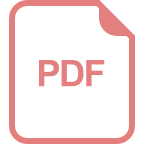
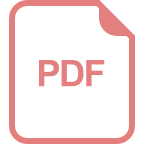
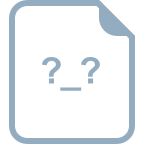
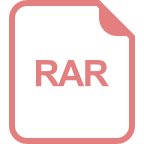
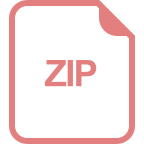
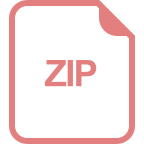
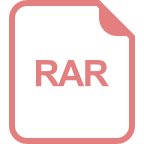
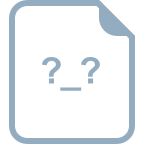
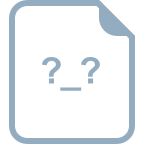
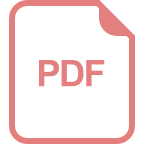
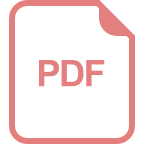
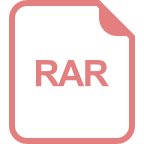




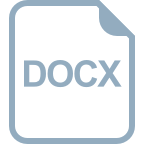