使用pulp创建多个订单,每个订单中有多个箱子匹配多种尺寸的箱子最小耗材的python代码
时间: 2024-03-10 14:50:40 浏览: 28
可以参考以下的Python代码来使用pulp创建多个订单,每个订单中有多个箱子匹配多种尺寸的箱子最小耗材问题:
```python
import pulp
class Order:
def __init__(self, order_id, boxes):
self.order_id = order_id
self.boxes = boxes
class Box:
def __init__(self, box_id, length, width, height, cost):
self.box_id = box_id
self.length = length
self.width = width
self.height = height
self.cost = cost
def create_order_matching_problem(orders, available_boxes):
# Initialize the optimization problem
problem = pulp.LpProblem("Order Matching Problem", pulp.LpMinimize)
# Define decision variables to represent the number of each box size to use for each order box
# Example: x[(1, 2, 3)] represents the number of the first available box size to use for the second order box
x = pulp.LpVariable.dicts("x", [(order.order_id, box.box_id) for order in orders for box in order.boxes],
lowBound=0, cat='Integer')
# Define the objective function to minimize the total cost of boxes used
problem += pulp.lpSum([x[(order.order_id, box.box_id)] * box.cost for order in orders for box in available_boxes])
# Define constraints to ensure that each order box is matched with at least one box size
for order in orders:
for box in order.boxes:
problem += pulp.lpSum([x[(order.order_id, available_box.box_id)] for available_box in available_boxes
if (available_box.length >= box.length and
available_box.width >= box.width and
available_box.height >= box.height)]) >= 1
# Define constraints to ensure that the total volume of boxes used for each order does not exceed the total volume of order boxes
for order in orders:
problem += pulp.lpSum([x[(order.order_id, available_box.box_id)] * available_box.length * available_box.width * available_box.height
for available_box in available_boxes]) <= pulp.lpSum([box.length * box.width * box.height for box in order.boxes])
return problem
# Example usage
orders = [
Order(1, [Box(1, 15, 15, 15, 10), Box(2, 25, 25, 25, 20), Box(3, 35, 35, 35, 30)]),
Order(2, [Box(1, 10, 10, 10, 5), Box(2, 20, 20, 20, 10), Box(3, 30, 30, 30, 15)]),
Order(3, [Box(1, 20, 20, 20, 15), Box(2, 30, 30, 30, 25), Box(3, 40, 40, 40, 35)])
]
available_boxes = [
Box(1, 10, 10, 10, 5),
Box(2, 20, 20, 20, 10),
Box(3, 30, 30, 30, 15)
]
problem = create_order_matching_problem(orders, available_boxes)
problem.solve()
# Print the optimal solution
for order in orders:
print(f"Order {order.order_id}:")
for box in order.boxes:
for available_box in available_boxes:
if x[(order.order_id, available_box.box_id)].value() > 0:
if (available_box.length >= box.length and
available_box.width >= box.width and
available_box.height >= box.height):
print(f"\t{int(x[(order.order_id, available_box.box_id)].value())} of box {available_box.box_id} (cost: {available_box.cost})")
print(f"Total cost: {int(pulp.value(problem.objective))}")
```
这个代码使用pulp库来定义一个线性规划问题,最小化使用的总箱子成本。在create_order_matching_problem函数中,我们定义了决策变量表示每个订单箱子使用的每种箱子尺寸的数量,并定义了目标函数最小化总成本。我们还定义了一些约束条件,以确保每个订单箱子至少匹配一个箱子尺寸,同时总体积不超过订单箱子的总体积。
在主函数中,我们创建了一些示例订单和可用箱子尺寸,并将它们传递给create_order_matching_problem函数,然后我们解决了线性规划问题,并打印出最优解,即每个订单箱子使用的每种箱子尺寸的数量以及总成本。
相关推荐
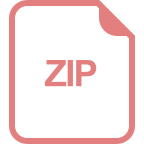
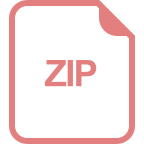
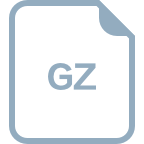














