文本文件中每一行是一个学生的成绩,读取每一行学生的信息,计算总分,按照总分进行排序,将排序后的学生信息写入另一个文本文件中。 c++ 使用动态数组
时间: 2024-02-24 11:54:52 浏览: 39
好的,根据你的要求,我可以提供以下的 C++ 代码实现,使用动态数组:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义学生信息结构体
struct Student {
string name; // 学生姓名
int score1; // 成绩1
int score2; // 成绩2
int score3; // 成绩3
int total; // 总分
// 构造函数
Student(string n, int s1, int s2, int s3) {
name = n;
score1 = s1;
score2 = s2;
score3 = s3;
total = s1 + s2 + s3;
}
};
// 比较函数,用于排序
bool cmp(Student a, Student b) {
return a.total > b.total;
}
int main() {
// 读取文件名
string filename;
cout << "请输入要读取的文件名:";
cin >> filename;
// 打开文件
ifstream fin(filename);
if (!fin.is_open()) {
cout << "无法打开文件 " << filename << endl;
return 0;
}
// 读取每一行学生信息
vector<Student> students;
string line;
while (getline(fin, line)) {
// 解析一行学生信息
string name;
int score1, score2, score3;
stringstream ss(line);
ss >> name >> score1 >> score2 >> score3;
// 创建学生对象并添加到学生信息列表中
students.push_back(Student(name, score1, score2, score3));
}
// 关闭文件
fin.close();
// 按照总分进行排序
sort(students.begin(), students.end(), cmp);
// 输出排序后的学生信息
for (int i = 0; i < students.size(); i++) {
cout << students[i].name << " "
<< students[i].score1 << " "
<< students[i].score2 << " "
<< students[i].score3 << " "
<< students[i].total << endl;
}
// 写入到另一个文件中
string output_filename;
cout << "请输入要写入的文件名:";
cin >> output_filename;
// 打开文件
ofstream fout(output_filename);
if (!fout.is_open()) {
cout << "无法打开文件 " << output_filename << endl;
return 0;
}
// 写入每一行学生信息
for (int i = 0; i < students.size(); i++) {
fout << students[i].name << " "
<< students[i].score1 << " "
<< students[i].score2 << " "
<< students[i].score3 << " "
<< students[i].total << endl;
}
// 关闭文件
fout.close();
// 程序结束
return 0;
}
```
在这个程序中,我们使用了一个动态数组 `vector` 来存储学生信息。在读取文件时,我们使用了 `stringstream` 类对一行字符串进行解析,得到每个学生的姓名和成绩,然后创建一个 `Student` 对象并添加到 `vector` 中。在排序时,我们使用了 `sort` 函数,并自定义了 `cmp` 函数作为比较函数。最后,我们使用 `ofstream` 类将排序后的学生信息写入到另一个文件中。
相关推荐
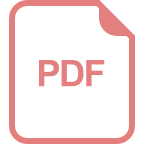
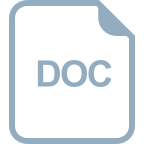
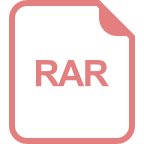
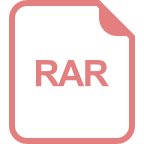
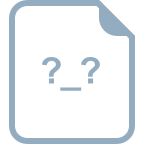
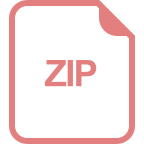
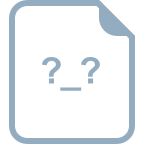
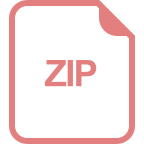
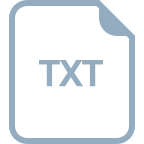
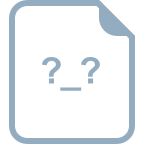