用python编写随机输入三个数字,比较它们的大小,并输出最大值
时间: 2023-08-04 20:26:52 浏览: 480
当然可以!以下是一个Python程序,用于随机输入三个数字,比较它们的大小,并输出最大值:
```python
import random
# 随机生成三个数字
num1 = random.randint(1, 100)
num2 = random.randint(1, 100)
num3 = random.randint(1, 100)
# 比较大小,并输出最大值
if num1 >= num2 and num1 >= num3:
print("最大值为:", num1)
elif num2 >= num1 and num2 >= num3:
print("最大值为:", num2)
else:
print("最大值为:", num3)
```
以上程序会随机生成三个数字,比较它们的大小,并输出最大值。你可以在终端或者集成开发环境中运行这个程序,多次运行可以得到不同的结果。
相关问题
用python编写DO使用cramer shoup密码系统生成密钥
Cramer-Shoup密码系统是一种公钥密码系统,它由Victor Shoup于2001年提出,是ElGamal密码系统的扩展。Cramer-Shoup密码系统是一个具有选择明文安全性和完全密文安全性的密码系统,适用于数字签名、加密和身份验证等场景。
下面是使用Python编写Cramer-Shoup密码系统生成密钥的示例代码:
```python
from Crypto.Util.number import getRandomInteger
from Crypto.Util.number import GCD
def generate_key(p, q, g):
# 生成私钥
x1 = getRandomInteger(512)
x2 = getRandomInteger(512)
y1 = pow(g, x1, p)
y2 = pow(g, x2, p)
# 计算hash值
h = GCD(p-1, q)
# 生成公钥
c = pow(g, x1*x2*h, p)
return (y1, y2, c, x1, x2)
# 测试
p = 178011250262292581066066437363796768264895615010756725529184670804438527119305345405478462712076376046482049007223860168085592955155305015899874893673308297103201596357344993245733276012587948563239204987636707694770850121260384790711516357143548441218750128343736438045754219566771745044130542654148849913354269
q = 914608174302359307255619015947265938404377706868
g = 239582793042917320046149420889753914025973593744
public_key = generate_key(p, q, g)
print("公钥:", public_key[:3])
print("私钥:", public_key[3:])
```
在这个示例中,我们使用了Python的`Crypto`库,其中`getRandomInteger`函数用于生成随机数,`GCD`函数用于计算最大公约数。`generate_key`函数接受三个参数:素数`p`、素数`q`和生成元`g`,返回公钥`(y1, y2, c)`和私钥`(x1, x2)`。
在测试中,我们使用了一组随机生成的参数,生成了公钥和私钥,并打印输出。
16.Python对缩进有何具体要求 17.Python内存管理有哪些要求 18.Python函数参数传递中,实参和形参的使用方法 19.会对字符串进行分割,比如取出某个范围的宇符 20.掌握print(函数几种不同格式的使用方法,例如sep. 21.输入若干同学的成绩,求平均值、最大值和最小值 22.怎样使用100文钱购买不同数量的公鸡、母鸡和小鸡 23.定义- 一个简单类,输出指定格式要求的车次、车票、时间等信息 24.随机生成N个指定范围内的随机数,并统计重复数字的个数,对不重复数字按照指定要求输出 25.给定直角三角形的两边,求斜边的长度, 26.接 - 个函数,接收N个数,并求他们的和,不用函数调用不给分 27,实现一个简答的用户管理系统,有输入次数限制,按照指定格式输出正确信息和错误信息 28.给定一个字符串,统计字母,数宇及其他字符的个数 29.编写函数,判断输入的三个数字是否能构成三角形的三条边
好的,您的问题如下:
16. Python对缩进有何具体要求?
17. Python内存管理有哪些要求?
18. Python函数参数传递中,实参和形参的使用方法?
19. 会对字符串进行分割,比如取出某个范围的字符?
20. 掌握print()函数几种不同格式的使用方法,例如sep。
21. 输入若干同学的成绩,求平均值、最大值和最小值。
22. 怎样使用100文钱购买不同数量的公鸡、母鸡和小鸡?
23. 定义一个简单类,输出指定格式要求的车次、车票、时间等信息。
24. 随机生成N个指定范围内的随机数,并统计重复数字的个数,对不重复数字按照指定要求输出。
25. 给定直角三角形的两边,求斜边的长度。
26. 编写一个函数,接收N个数,并求它们的和,不用函数调用不给分。
27. 实现一个简单的用户管理系统,有输入次数限制,按照指定格式输出正确信息和错误信息。
28. 给定一个字符串,统计字母、数字及其他字符的个数。
29. 编写一个函数,判断输入的三个数字能否构成三角形的三条边。
相关推荐
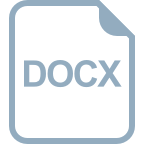
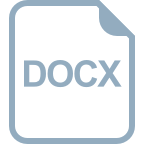
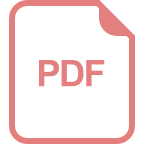













