将链表逆置,即最后一个结点变成第一个结点,原来倒数第二个结点变成第二个结点,如此等等。
时间: 2023-06-01 10:03:31 浏览: 52
以下是实现链表逆置的Python代码:
```python
class Node:
def __init__(self, data=None, next=None):
self.data = data
self.next = next
class LinkedList:
def __init__(self):
self.head = None
def add(self, data):
node = Node(data)
if self.head is None:
self.head = node
return
current = self.head
while current.next is not None:
current = current.next
current.next = node
def reverse(self):
previous = None
current = self.head
while current is not None:
next_node = current.next
current.next = previous
previous = current
current = next_node
self.head = previous
def display(self):
current = self.head
while current is not None:
print(current.data, end=" ")
current = current.next
print()
# 测试代码
if __name__ == '__main__':
linked_list = LinkedList()
linked_list.add(1)
linked_list.add(2)
linked_list.add(3)
linked_list.add(4)
linked_list.add(5)
linked_list.display()
linked_list.reverse()
linked_list.display()
```
在上面的代码中,我们定义了一个`Node`类来表示链表中的每个结点,以及一个`LinkedList`类来表示整个链表。在`LinkedList`类中,我们定义了`add`方法用于向链表中添加新的结点,`reverse`方法用于将链表逆置,以及`display`方法用于打印链表的所有结点。
具体来说,`reverse`方法中我们使用了三个指针:`current`指向当前结点,`previous`指向前一个结点,`next_node`指向下一个结点。我们不断地将`current`结点的`next`指针指向`previous`结点,然后将`previous`和`current`向右移动一个结点,直到`current`变成了`None`,也就是链表的末尾。最后,我们将链表的头结点指向逆置后的第一个结点,即`previous`。
在测试代码中,我们首先构建了一个包含五个结点的链表,然后打印了链表的所有结点。接着,我们调用了`reverse`方法将链表逆置,并再次打印了链表的所有结点,可以看到链表已经逆置成功。
相关推荐
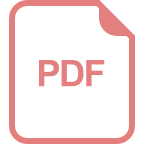
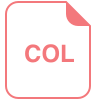
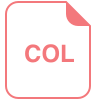
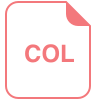
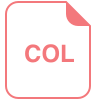
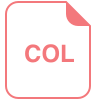








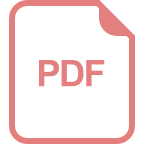