实现线性表的链式存储定义,完成单链表的创建(头插法、尾插法)、插入、删除、查找等常用操作,将线性链表逆置,即最后一个结点变成第一个结点,原来倒数第二个结点变成第二个结点,以此类推。实现c语音代码
时间: 2023-10-19 21:12:57 浏览: 44
以下是基于指针实现的单链表的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义单链表的节点结构体 */
typedef struct ListNode {
int val; // 节点的值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
/* 头插法创建单链表 */
ListNode* createListByHeadInsert(int* nums, int numsSize) {
ListNode* head = NULL; // 头节点
ListNode* node; // 新节点
for (int i = 0; i < numsSize; i++) {
node = (ListNode*)malloc(sizeof(ListNode)); // 申请新节点
node->val = nums[i];
node->next = head; // 新节点指向原头节点
head = node; // 更新头节点
}
return head;
}
/* 尾插法创建单链表 */
ListNode* createListByTailInsert(int* nums, int numsSize) {
ListNode* head = NULL; // 头节点
ListNode* tail = NULL; // 尾节点
ListNode* node; // 新节点
for (int i = 0; i < numsSize; i++) {
node = (ListNode*)malloc(sizeof(ListNode)); // 申请新节点
node->val = nums[i];
node->next = NULL; // 新节点指向NULL
if (tail == NULL) { // 第一个节点
head = node;
tail = node;
} else {
tail->next = node; // 尾节点指向新节点
tail = node; // 更新尾节点
}
}
return head;
}
/* 遍历单链表 */
void traverseList(ListNode* head) {
ListNode* p = head;
while (p != NULL) {
printf("%d->", p->val);
p = p->next;
}
printf("NULL\n");
}
/* 插入节点 */
ListNode* insertListNode(ListNode* head, int val, int index) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode)); // 申请新节点
node->val = val;
if (index == 0) { // 插入头节点
node->next = head;
head = node;
} else {
ListNode* p = head;
for (int i = 0; i < index - 1; i++) { // 找到待插入位置的前一个节点
p = p->next;
}
node->next = p->next; // 新节点指向原节点
p->next = node; // 前一个节点指向新节点
}
return head;
}
/* 删除节点 */
ListNode* deleteListNode(ListNode* head, int index) {
if (index == 0) { // 删除头节点
ListNode* p = head;
head = head->next;
free(p);
} else {
ListNode* p = head;
for (int i = 0; i < index - 1; i++) { // 找到待删除位置的前一个节点
p = p->next;
}
ListNode* q = p->next; // 待删除节点
p->next = q->next; // 前一个节点指向下一个节点
free(q);
}
return head;
}
/* 查找节点 */
int findListNode(ListNode* head, int val) {
ListNode* p = head;
int index = 0;
while (p != NULL) {
if (p->val == val) {
return index;
}
p = p->next;
index++;
}
return -1; // 没有找到
}
/* 单链表逆置 */
ListNode* reverseList(ListNode* head) {
ListNode* prev = NULL; // 前一个节点
ListNode* curr = head; // 当前节点
ListNode* next = NULL; // 下一个节点
while (curr != NULL) {
next = curr->next; // 先保存下一个节点
curr->next = prev; // 当前节点指向前一个节点
prev = curr; // 更新前一个节点
curr = next; // 更新当前节点
}
return prev; // 返回新的头节点
}
int main() {
int nums[] = {1, 2, 3, 4, 5};
int numsSize = sizeof(nums) / sizeof(int);
ListNode* head1 = createListByHeadInsert(nums, numsSize);
printf("Head insert: ");
traverseList(head1);
ListNode* head2 = createListByTailInsert(nums, numsSize);
printf("Tail insert: ");
traverseList(head2);
head2 = insertListNode(head2, 6, 3);
printf("Insert 6 at index 3: ");
traverseList(head2);
head2 = deleteListNode(head2, 4);
printf("Delete node at index 4: ");
traverseList(head2);
int index = findListNode(head2, 2);
if (index != -1) {
printf("The index of node with value 2: %d\n", index);
} else {
printf("Node with value 2 not found.\n");
}
head2 = reverseList(head2);
printf("Reversed list: ");
traverseList(head2);
return 0;
}
```
相关推荐
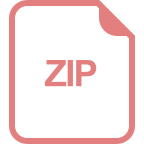
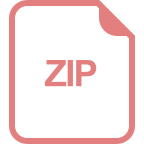











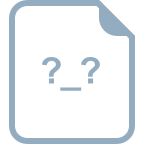
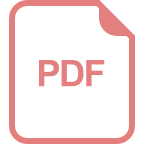
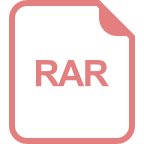