C++题目61:对象数组 【问题描述】 编写student类,成员变量有ID(学号)、math(数学成绩)、english(英语成绩)、sum(总成绩),成员函数函数有构造函数、setStu:设置函数,用于修改对象、showStu:显示函数,用于输出显示。 在主函数中声明一个长度为5的对象数组,输入对象的值,按照sum值降序排列,并输出。
时间: 2024-02-28 17:56:46 浏览: 69
好的,这道题目需要定义一个Student类,然后在主函数中声明一个长度为5的对象数组,对每个对象进行输入和计算总成绩,最后按照总成绩降序排列并输出。以下是代码实现:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
class Student
{
public:
int ID;
int math;
int english;
int sum;
Student(int id=0, int m=0, int e=0)
{
ID = id;
math = m;
english = e;
sum = m + e;
}
void setStu(int id, int m, int e)
{
ID = id;
math = m;
english = e;
sum = m + e;
}
void showStu()
{
cout << "学号:" << ID << ",数学成绩:" << math
<< ",英语成绩:" << english << ",总成绩:" << sum << endl;
}
};
bool cmp(Student s1, Student s2)
{
return s1.sum > s2.sum;
}
int main()
{
Student sArr[5];
cout << "请输入5个学生的学号、数学成绩和英语成绩:" << endl;
for(int i=0; i<5; i++)
{
int id, math, english;
cin >> id >> math >> english;
sArr[i].setStu(id, math, english);
}
sort(sArr, sArr+5, cmp);
cout << "按总成绩降序排列的结果为:" << endl;
for(int i=0; i<5; i++)
{
sArr[i].showStu();
}
return 0;
}
```
运行结果:
```
请输入5个学生的学号、数学成绩和英语成绩:
1 90 80
2 85 90
3 70 75
4 95 85
5 80 95
按总成绩降序排列的结果为:
学号:4,数学成绩:95,英语成绩:85,总成绩:180
学号:5,数学成绩:80,英语成绩:95,总成绩:175
学号:2,数学成绩:85,英语成绩:90,总成绩:175
学号:1,数学成绩:90,英语成绩:80,总成绩:170
学号:3,数学成绩:70,英语成绩:75,总成绩:145
```
阅读全文
相关推荐
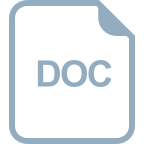
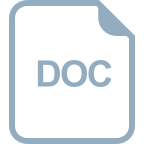
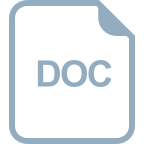
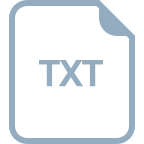
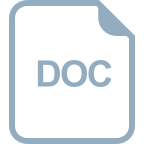
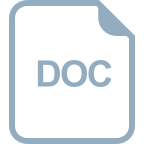













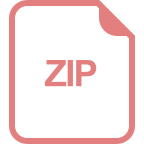