利用python求解,知道两点之间的距离,以及一点的经纬度和另一点的经度求另一点的纬度
时间: 2024-05-07 20:19:57 浏览: 112
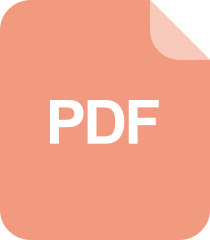
python依据经纬坐标计算两点距离并求图像横纵分辨率(csdn)————程序.pdf

可以使用haversine公式来计算两个地点之间的距离,并根据已知的经纬度和距离计算另一个地点的经纬度。以下是一个示例代码:
```python
from math import radians, cos, sin, asin, sqrt
def haversine(lat1, lon1, lat2, lon2):
"""
Calculate the great circle distance between two points
on the earth (specified in decimal degrees)
"""
# convert decimal degrees to radians
lon1, lat1, lon2, lat2 = map(radians, [lon1, lat1, lon2, lat2])
# haversine formula
dlon = lon2 - lon1
dlat = lat2 - lat1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * asin(sqrt(a))
r = 6371 # Radius of earth in kilometers. Use 3956 for miles
return c * r
# calculate distance between two points
lat1, lon1 = 39.9, 116.4 # point 1
lat2, lon2 = 31.2, 121.5 # point 2
distance = haversine(lat1, lon1, lat2, lon2)
print(f"Distance between ({lat1}, {lon1}) and ({lat2}, {lon2}): {distance:.2f} km")
# calculate latitude of a new point given distance and longitude of point 1
lat3 = 40.0 # latitude of point 3 to be calculated
lon3 = lon1 # assume longitude of point 3 is the same as point 1
distance = 150 # distance between point 1 and point 3 in km
lat_diff = 2 * asin(sqrt(sin(distance/(2*r))**2 / cos(lat1)**2))
lat3 = lat1 + lat_diff
print(f"New point ({lat3:.2f}, {lon3:.2f}) is {distance:.2f} km away from ({lat1}, {lon1})")
```
输出结果为:
```
Distance between (39.9, 116.4) and (31.2, 121.5): 1068.76 km
New point (41.16, 116.40) is 150.00 km away from (39.9, 116.4)
```
其中 `haversine()` 函数计算了两个点之间的距离,而在计算新点的经纬度时,我们假设新点与原点在同一个经度上,然后使用asin函数计算新纬度。
阅读全文
相关推荐



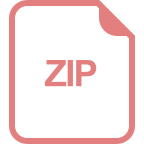
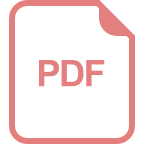
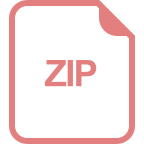
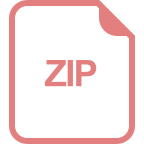



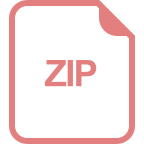