python实现生产者消费者多线程程序,三个生产者
时间: 2023-11-17 11:02:06 浏览: 122
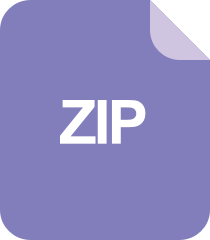
生产者消费者多线程程序
和三个消费者,可以使用Python中的`queue`模块来实现。具体实现如下:
```python
import queue
import threading
import time
# 创建一个线程安全的队列
q = queue.Queue()
# 生产者线程函数
def producer():
for i in range(10):
# 将生产的物品放入队列中
q.put(f'product_{i}')
print(f'Producer {threading.current_thread().name} produced product_{i}')
time.sleep(1)
# 消费者线程函数
def consumer():
while True:
# 从队列中取出物品
item = q.get()
if item is None:
break
print(f'Consumer {threading.current_thread().name} consumed {item}')
time.sleep(1)
# 创建3个生产者线程
for i in range(3):
t = threading.Thread(target=producer, name=f'Producer_{i}')
t.start()
# 创建3个消费者线程
for i in range(3):
t = threading.Thread(target=consumer, name=f'Consumer_{i}')
t.start()
# 等待所有生产者线程结束
for i in range(3):
q.put(None)
# 等待所有线程结束
for t in threading.enumerate():
if t != threading.current_thread():
t.join()
```
在这个例子中,我们创建了一个线程安全的队列来存储生产者生产的物品,每个生产者线程都会往队列中放入10个物品,每个消费者线程都会从队列中取出物品并消费。为了让消费者线程能够在队列为空时等待生产者生产物品,我们使用了队列的默认阻塞行为。当队列为空时,`get()`方法会一直阻塞,直到队列中有物品为止。同理,当队列满时,`put()`方法也会阻塞。
最后,我们使用`None`来表示生产者已经生产完毕,消费者线程在取出`None`时就会退出循环,从而结束线程。
阅读全文
相关推荐
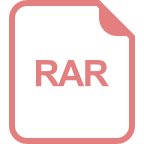
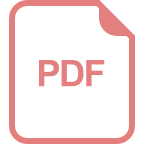
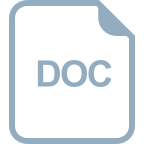
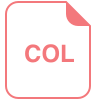









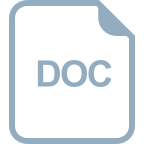
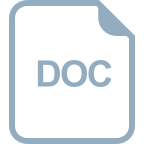