c语言编程实现如下功能: ①建立索引查找表; ②利用索引查找确定给定记录在索引查找表中的块号和在块中的位置。 索引查找表有索引表和块表两部分所构成,其中索引表存储的是各块记录中的最大关键字值和各块的起始存储地址,用顺序存储结构,各块的起始存储地址的初始值置为空指针;而块表中存储的是查找表中的所有记录并且按块有序,用链式存储或顺序存储结构,在此用链式存储结构。
时间: 2024-03-26 10:35:33 浏览: 191
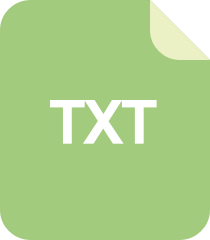
链表的基本操作(字符型数据).txt
以下是实现功能的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义索引表结构体
typedef struct {
int maxKey; // 块内最大关键字
int start; // 块的起始地址
} indexItem;
// 定义块表结构体
typedef struct {
int key; // 关键字
int next; // 下一个元素的位置
} blockItem;
// 建立索引查找表
void createIndexTable(indexItem indexTable[], blockItem blockTable[], int n, int blockSize) {
int index = 0; // 当前索引表位置
int block = 0; // 当前块表位置
int maxKey = 0; // 当前块内最大关键字
indexTable[index].start = 0; // 第一个块的起始地址为0
for(int i = 0; i < n; i++) {
printf("请输入第%d个记录的关键字:", i+1);
scanf("%d", &blockTable[block].key);
if(blockTable[block].key > maxKey) {
maxKey = blockTable[block].key;
}
block++;
if(block % blockSize == 0) { // 块已满,需要新建块
indexTable[index].maxKey = maxKey; // 将当前块内最大关键字存入索引表
maxKey = 0; // 重置当前块内最大关键字
indexTable[++index].start = block; // 新建块的起始地址为当前块的下一个位置
}
}
if(block % blockSize != 0) { // 最后一个块未满,需要将其最大关键字存入索引表
indexTable[index].maxKey = maxKey;
}
}
// 索引查找
void indexSearch(indexItem indexTable[], blockItem blockTable[], int n, int blockSize, int x) {
int index = 0;
while(index < n && x > indexTable[index].maxKey) { // 在索引表中查找块号
index++;
}
if(index == n) { // 未找到块号
printf("未找到该记录!\n");
return;
}
int block = indexTable[index].start; // 块起始位置
while(block < n && block - indexTable[index].start < blockSize && blockTable[block].key != x) { // 在当前块中查找记录
block = blockTable[block].next;
}
if(block >= n || block - indexTable[index].start >= blockSize) { // 未找到记录
printf("未找到该记录!\n");
return;
}
printf("该记录位于第%d块第%d个位置。\n", index+1, block-indexTable[index].start+1);
}
int main() {
int n, blockSize, x;
printf("请输入查找表中记录的个数:");
scanf("%d", &n);
printf("请输入每个块的大小:");
scanf("%d", &blockSize);
blockItem *blockTable = (blockItem*)malloc(n * sizeof(blockItem)); // 动态分配块表空间
indexItem *indexTable = (indexItem*)malloc((n/blockSize + 1) * sizeof(indexItem)); // 动态分配索引表空间
createIndexTable(indexTable, blockTable, n, blockSize); // 建立索引查找表
while(1) {
printf("请输入要查找的记录的关键字(输入-1退出):");
scanf("%d", &x);
if(x == -1) {
break;
}
indexSearch(indexTable, blockTable, n, blockSize, x); // 索引查找
}
free(blockTable); // 释放块表空间
free(indexTable); // 释放索引表空间
return 0;
}
```
阅读全文
相关推荐
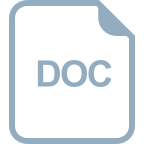
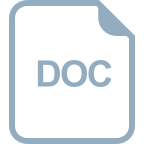
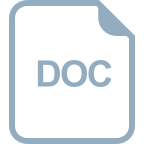
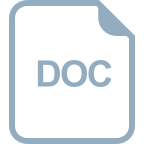
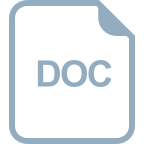
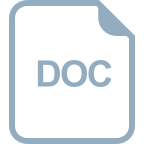
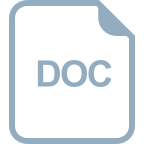
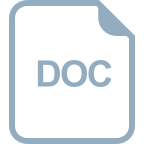

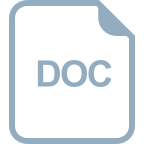
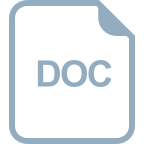
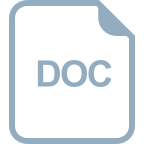
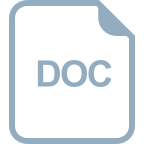
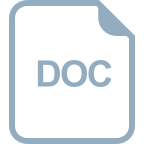
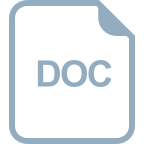
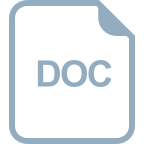

