使用c++编写一个mfc截图功能,并且图片保存为.png格式
时间: 2024-02-27 17:51:22 浏览: 29
好的,下面是一个简单的C++ MFC截图功能的实现,其中使用了GDI+库来保存为.png格式的图片:
```
#include <afxwin.h>
#include <gdiplus.h> // 需要添加GDI+库的引用
using namespace Gdiplus;
class CScreenshotDlg : public CDialogEx
{
public:
CScreenshotDlg(CWnd* pParent = nullptr) : CDialogEx(IDD_SCREENSHOT_DIALOG, pParent) {}
protected:
virtual void DoDataExchange(CDataExchange* pDX) override { CDialogEx::DoDataExchange(pDX); }
virtual BOOL OnInitDialog() override;
afx_msg void OnCaptureButtonClicked();
DECLARE_MESSAGE_MAP()
private:
CWnd* m_pParentWnd;
};
BEGIN_MESSAGE_MAP(CScreenshotDlg, CDialogEx)
ON_BN_CLICKED(IDC_CAPTURE_BUTTON, &CScreenshotDlg::OnCaptureButtonClicked)
END_MESSAGE_MAP()
BOOL CScreenshotDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
m_pParentWnd = GetDesktopWindow();
return TRUE;
}
void CScreenshotDlg::OnCaptureButtonClicked()
{
CRect rect;
m_pParentWnd->GetWindowRect(rect);
CDC* pDC = m_pParentWnd->GetDC();
CDC memDC;
memDC.CreateCompatibleDC(pDC);
CBitmap bmp;
bmp.CreateCompatibleBitmap(pDC, rect.Width(), rect.Height());
memDC.SelectObject(&bmp);
memDC.BitBlt(0, 0, rect.Width(), rect.Height(), pDC, 0, 0, SRCCOPY);
GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
CLSID encoderClsid;
GetEncoderClsid(L"image/png", &encoderClsid);
Image image(bmp);
image.Save(L"capture.png", &encoderClsid, NULL);
GdiplusShutdown(gdiplusToken);
ReleaseDC(pDC);
memDC.DeleteDC();
}
int main()
{
AfxWinInit(::GetModuleHandle(NULL), NULL, ::GetCommandLine(), 0);
CScreenshotDlg dlg;
dlg.DoModal();
return 0;
}
```
其中,GetEncoderClsid函数是获取.png格式编码器的CLSID的函数,实现如下:
```
int GetEncoderClsid(const WCHAR* format, CLSID* pClsid)
{
UINT num = 0;
UINT size = 0;
ImageCodecInfo* pImageCodecInfo = NULL;
GetImageEncodersSize(&num, &size);
if (size == 0)
return -1;
pImageCodecInfo = (ImageCodecInfo*)(malloc(size));
if (pImageCodecInfo == NULL)
return -1;
GetImageEncoders(num, size, pImageCodecInfo);
for (UINT i = 0; i < num; ++i)
{
if (wcscmp(pImageCodecInfo[i].MimeType, format) == 0)
{
*pClsid = pImageCodecInfo[i].Clsid;
free(pImageCodecInfo);
return i;
}
}
free(pImageCodecInfo);
return -1;
}
```
这个实现中,我们首先获取了父窗口的大小和DC,然后创建了一个兼容DC和位图,并将父窗口的图像复制到位图中。接着,我们使用GDI+库创建了一个Image对象,并使用Save函数将其保存为.png格式的图像。注意,该函数需要传入.png格式的编码器的CLSID,因此我们使用了GetEncoderClsid函数获取该编码器的CLSID。最后,我们释放了DC和位图,关闭了GDI+库。
希望这个实现对你有所帮助!
相关推荐
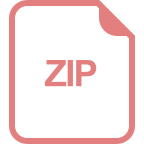
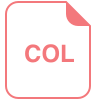













