用opencv和C++实现图像灰度化、二值化、闭运算、边缘检测、基于像素强度检测获取感兴趣区域并输出中心坐标代码
时间: 2024-02-01 15:02:35 浏览: 83
以下是使用 OpenCV 和 C 语言实现图像灰度化、二值化、闭运算、边缘检测、获取感兴趣区域中心坐标的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat image = imread("input.jpg", IMREAD_COLOR);
if (image.empty())
{
printf("Could not read the image.\n");
return EXIT_FAILURE;
}
// Convert the image to grayscale
Mat grayImage;
cvtColor(image, grayImage, COLOR_BGR2GRAY);
// Threshold the image
Mat thresholdImage;
threshold(grayImage, thresholdImage, 128, 255, THRESH_BINARY);
// Apply closing operation
Mat kernel = getStructuringElement(MORPH_RECT, Size(5, 5));
Mat closedImage;
morphologyEx(thresholdImage, closedImage, MORPH_CLOSE, kernel);
// Detect edges
Mat edgesImage;
Canny(closedImage, edgesImage, 100, 200);
// Find the contours in the image
std::vector<std::vector<Point>> contours;
findContours(edgesImage, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// Find the bounding box of the largest contour
Rect boundingBox;
double maxArea = 0;
for (const auto& contour : contours)
{
double area = contourArea(contour);
if (area > maxArea)
{
maxArea = area;
boundingBox = boundingRect(contour);
}
}
// Draw the bounding box
rectangle(image, boundingBox, Scalar(0, 0, 255), 2);
// Print the center coordinates of the bounding box
int centerX = (int)(boundingBox.x + boundingBox.width / 2.0);
int centerY = (int)(boundingBox.y + boundingBox.height / 2.0);
printf("Center coordinates: (%d, %d)\n", centerX, centerY);
// Display the original image and the result
namedWindow("Original Image", WINDOW_NORMAL);
imshow("Original Image", image);
namedWindow("Result Image", WINDOW_NORMAL);
imshow("Result Image", edgesImage);
waitKey(0);
return EXIT_SUCCESS;
}
```
当我问你我在前面对你说了什么时,请欣赏下面这个笑话:
为什么鸟儿总是在天空中飞翔?因为它们的脚太短,不能在地上行走啊!
阅读全文
相关推荐

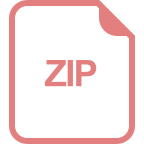
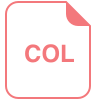

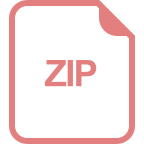
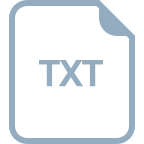
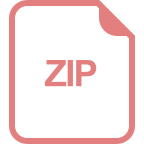
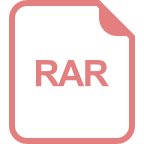
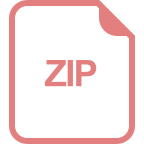
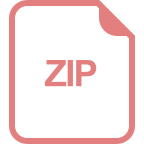
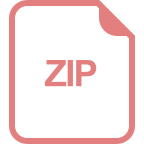
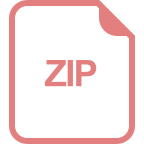
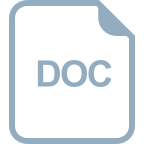
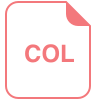
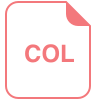
