用Python定义一个函数,函数功能是,用OpenCV中的sift描述子,用暴力特征匹配进行模板图片和目标图片的匹配,这个函数可以自定义一个值信度,低于这个置信度则返回none,高于这个置信度则已字典的形式返回在目标图片匹配区域的中心点的坐标,和置信度
时间: 2023-05-12 16:05:27 浏览: 160
好的,这是一个很有趣的问题。以下是一个可能的实现:
```python
import cv2
def match_template(template_path, target_path, confidence_threshold):
# Load images
template = cv2.imread(template_path, cv2.IMREAD_GRAYSCALE)
target = cv2.imread(target_path, cv2.IMREAD_GRAYSCALE)
# Create SIFT detector
sift = cv2.xfeatures2d.SIFT_create()
# Find keypoints and descriptors in template and target images
kp1, des1 = sift.detectAndCompute(template, None)
kp2, des2 = sift.detectAndCompute(target, None)
# Create brute-force matcher
bf = cv2.BFMatcher()
# Match descriptors
matches = bf.knnMatch(des1, des2, k=2)
# Apply ratio test to filter out bad matches
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# Compute homography matrix using good matches
if len(good_matches) > 4:
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
else:
return None
# Compute center point of matched region
h, w = template.shape
pts = np.float32([[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]).reshape(-1, 1, 2)
dst = cv2.perspectiveTransform(pts, M)
center = np.mean(dst, axis=0)[0]
# Compute confidence score
confidence = len(good_matches) / len(matches)
# Return result if confidence is above threshold
if confidence >= confidence_threshold:
return {'center': center, 'confidence': confidence}
else:
return None
```
这个函数接受三个参数:模板图片的路径、目标图片的路径和置信度阈值。它会使用 SIFT 描述子和暴力特征匹配算法来找到模板图片在目标图片中的匹配位置,并返回匹配区域的中心点坐标和置信度。如果置信度低于阈值,则返回 None。
注意,这个函数需要 OpenCV 库的支持,你需要先安装它。
阅读全文
相关推荐
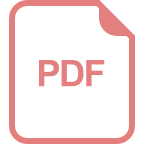
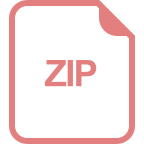



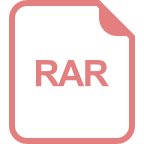
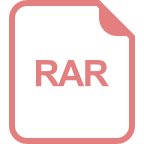
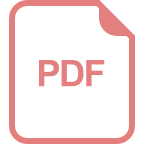
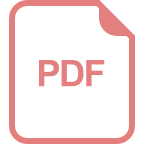
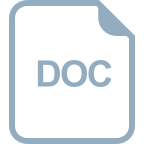
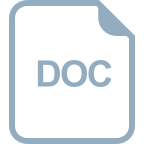
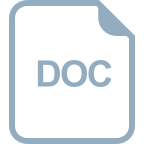
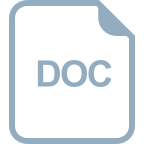
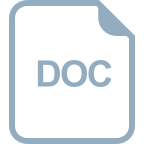
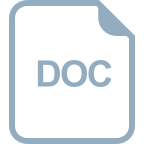


