java Apache sshd断线重连
时间: 2024-04-30 11:19:26 浏览: 288
Apache SSHD 提供了重连功能的支持,可以在连接中断后自动重连。下面是一个示例代码:
```java
public class SshClient {
private static final Logger LOGGER = LoggerFactory.getLogger(SshClient.class);
private final String host;
private final int port;
private final String username;
private final String password;
private SshClientSession sshClientSession;
private boolean connected = false;
public SshClient(String host, int port, String username, String password) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
}
public void connect() throws IOException, InterruptedException {
SshClient client = SshClient.setUpDefaultClient();
client.start();
ConnectFuture future = client.connect(host, port);
future.await();
sshClientSession = future.getSession();
sshClientSession.addPasswordIdentity(password);
sshClientSession.auth().verify();
connected = true;
}
public void disconnect() throws IOException {
if (sshClientSession != null) {
sshClientSession.disconnect();
}
connected = false;
}
public boolean isConnected() {
return connected;
}
public SshClientSession getSshClientSession() {
return sshClientSession;
}
public static void main(String[] args) throws IOException, InterruptedException {
SshClient sshClient = new SshClient("localhost", 22, "username", "password");
sshClient.connect();
SshClientSession sshClientSession = sshClient.getSshClientSession();
sshClientSession.addCloseFutureListener(new SshFutureListener<CloseFuture>() {
@Override
public void operationComplete(CloseFuture future) {
LOGGER.info("Session closed, trying to reconnect...");
while (true) {
try {
sshClient.connect();
LOGGER.info("Reconnected successfully.");
break;
} catch (IOException | InterruptedException e) {
LOGGER.info("Failed to reconnect. Retrying in 5 seconds...");
}
Thread.sleep(5000);
}
}
});
while (sshClient.isConnected()) {
// Do something with the SSH session
}
sshClient.disconnect();
}
}
```
在 `main()` 方法中,我们首先创建了一个 SSH 客户端 `SshClient`,并通过 `connect()` 方法连接到 SSH 服务器。然后,我们获取到 SSH 会话 `SshClientSession`,并为其添加一个关闭监听器 `SshFutureListener`。当会话关闭时,我们在监听器中尝试重新连接 SSH 服务器。如果连接失败,我们将在 5 秒钟后重试,直到连接成功为止。
在 `while (sshClient.isConnected())` 循环中,我们可以执行一些操作,例如发送命令到 SSH 服务器,或者接收来自 SSH 服务器的响应。当连接断开时,循环将退出,然后我们通过 `disconnect()` 方法关闭 SSH 客户端。
需要注意的是,上述代码中的重连逻辑仅针对 SSH 会话断开的情况。如果 SSH 客户端与 SSH 服务器之间的 TCP 连接断开,那么需要使用其他方法进行重连,例如通过 TCP Keep-Alive 或者使用一个专门的 TCP 连接池等。
阅读全文
相关推荐
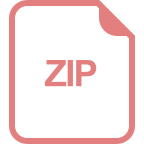
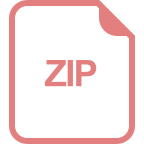

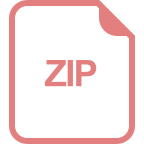
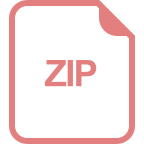
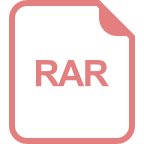
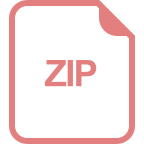
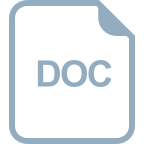






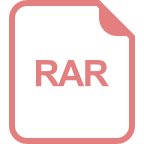

