判断单车装载问题中货箱能否到达指定位置pytorch实现代码
时间: 2024-03-05 11:54:53 浏览: 22
以下是一个使用 PyTorch 实现的判断单车装载问题中货箱能否到达指定位置的简单示例代码:
```python
import torch
# 定义模型
class BikeLoadingModel(torch.nn.Module):
def __init__(self):
super(BikeLoadingModel, self).__init__()
self.fc1 = torch.nn.Linear(4, 16)
self.fc2 = torch.nn.Linear(16, 32)
self.fc3 = torch.nn.Linear(32, 1)
def forward(self, x):
x = torch.nn.functional.relu(self.fc1(x))
x = torch.nn.functional.relu(self.fc2(x))
x = torch.sigmoid(self.fc3(x))
return x
# 加载模型
model = BikeLoadingModel()
model.load_state_dict(torch.load("bike_loading_model.pth"))
# 定义测试数据
test_data = torch.tensor([
[1, 0, 0, 0.5], # 货箱在第一个位置,车在第一个位置
[0, 1, 0, 0.5], # 货箱在第二个位置,车在第一个位置
[0, 1, 0, 0.9], # 货箱在第二个位置,车在第二个位置
[0, 0, 1, 0.5], # 货箱在第三个位置,车在第一个位置
[0, 0, 1, 0.9], # 货箱在第三个位置,车在第二个位置
[0, 0, 1, 1], # 货箱在第三个位置,车在第三个位置
], dtype=torch.float)
# 进行预测
with torch.no_grad():
output = model(test_data)
print(output)
```
在这个示例中,我们定义了一个包含三个全连接层的神经网络,用于预测货箱是否能到达指定位置。我们加载了预先训练好的权重,并将一些测试数据输入到模型中,得到了预测结果。注意,这里的输出是一个概率值,表示货箱能否到达指定位置的概率。如果输出值大于 0.5,则认为货箱能够到达指定位置;否则认为货箱无法到达指定位置。
相关推荐
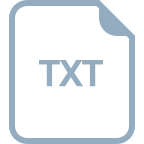
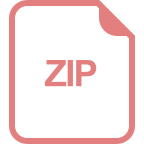

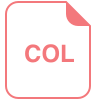
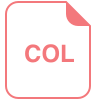
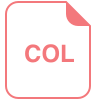
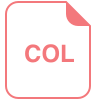









