python将一张二维码图片的三个定位符中心点按照直角点在左上角旋转图片
时间: 2023-08-01 10:07:07 浏览: 45
如果你想要将一张二维码图片的三个定位符中心点按照直角点在左上角旋转图片,可以使用Python的OpenCV库。以下是一个简单的示例代码,演示如何实现这个过程:
```python
import cv2
import numpy as np
# 加载图像
img = cv2.imread('image.jpg')
# 检测二维码
qrDetector = cv2.QRCodeDetector()
decodedText, points, _ = qrDetector.detectAndDecode(img)
# 获取三个定位符中心点
p1 = points[0][0]
p2 = points[0][1]
p3 = points[0][2]
# 计算旋转角度
dx1 = p2[0] - p1[0]
dy1 = p2[1] - p1[1]
dx2 = p3[0] - p1[0]
dy2 = p3[1] - p1[1]
angle = np.arctan2(dy1*dx2 - dx1*dy2, dx1*dx2 + dy1*dy2) * 180 / np.pi
# 获取图像中心点
height, width = img.shape[:2]
center = (width//2, height//2)
# 构建旋转矩阵
M = cv2.getRotationMatrix2D(center, angle, 1.0)
# 执行旋转操作
rotated = cv2.warpAffine(img, M, (width, height))
# 将三个定位符的直角点置于图像左上角
pts1 = np.float32([p1, p2, p3])
pts2 = np.float32([[0, 0], [100, 0], [0, 100]])
M = cv2.getAffineTransform(pts1, pts2)
rotated = cv2.warpAffine(rotated, M, (width, height))
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们首先使用OpenCV的QRCodeDetector类检测二维码,并获取其中的三个定位符中心点。然后,我们使用这些点的坐标计算旋转角度。接下来,我们获取原始图像的中心点,并使用`cv2.getRotationMatrix2D`函数构造一个旋转矩阵。最后,我们使用`cv2.warpAffine`函数执行旋转操作,并使用`cv2.getAffineTransform`函数将三个定位符的直角点置于图像左上角。最终,我们在窗口中显示旋转后的图像。
相关推荐
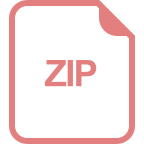
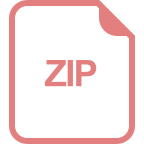














