生成BiFPN结构热力图的代码
时间: 2023-07-13 08:23:57 浏览: 80
下面是使用Python和TensorFlow实现生成BiFPN结构热力图的代码示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, UpSampling2D, concatenate, BatchNormalization, Activation
from tensorflow.keras.models import Model
from tensorflow.keras.utils import plot_model
import numpy as np
def build_bifpn(input_shape, num_channels, num_layers, num_filters):
# 定义输入层
inputs = Input(input_shape)
# 定义第一层
conv1 = Conv2D(num_filters, (3,3), padding='same', activation='relu')(inputs)
pool1 = MaxPooling2D(pool_size=(2,2))(conv1)
# 定义多层bifpn
layers = [pool1]
for i in range(num_layers):
# 前向传播
conv = Conv2D(num_filters, (3,3), padding='same', activation='relu')(layers[-1])
up = UpSampling2D(size=(2,2))(conv)
merge = concatenate([layers[-2], up], axis=3)
conv1 = Conv2D(num_filters, (3,3), padding='same', activation='relu')(merge)
conv2 = Conv2D(num_filters, (1,1), padding='same')(conv1)
bn = BatchNormalization()(conv2)
activation = Activation('relu')(bn)
# 添加到层列表中
layers.append(activation)
# 定义输出层
output = layers[-1]
output = Conv2D(num_channels, (1,1), padding='same', activation='sigmoid')(output)
# 构建模型
model = Model(inputs=[inputs], outputs=[output])
return model
# 定义输入形状,通道数和层数
input_shape = (128, 128, 3)
num_channels = 1
num_layers = 3
num_filters = 64
# 构建模型
model = build_bifpn(input_shape, num_channels, num_layers, num_filters)
# 绘制模型结构图
plot_model(model, to_file='bifpn.png', show_shapes=True)
# 生成热力图
from tensorflow.keras.utils import plot_model
import matplotlib.pyplot as plt
layer_names = []
for layer in model.layers:
layer_names.append(layer.name)
activations = model.predict(np.random.rand(1, 128, 128, 3))
images_per_row = 16
for layer_name, layer_activation in zip(layer_names, activations):
n_features = layer_activation.shape[-1]
size = layer_activation.shape[1]
n_cols = n_features // images_per_row
display_grid = np.zeros((size * n_cols, images_per_row * size))
for col in range(n_cols):
for row in range(images_per_row):
channel_image = layer_activation[0, :, :, col * images_per_row + row]
channel_image -= channel_image.mean()
channel_image /= channel_image.std()
channel_image *= 64
channel_image += 128
channel_image = np.clip(channel_image, 0, 255).astype('uint8')
display_grid[col * size : (col + 1) * size, row * size : (row + 1) * size] = channel_image
scale = 1. / size
plt.figure(figsize=(scale * display_grid.shape[1], scale * display_grid.shape[0]))
plt.title(layer_name)
plt.grid(False)
plt.imshow(display_grid, aspect='auto', cmap='viridis')
plt.show()
```
上述代码中使用了TensorFlow的keras API来构建BiFPN模型,并通过plot_model函数将模型结构绘制成图形化的png文件。使用模型的predict函数获取模型的输出,然后通过循环遍历每一层的输出,将其可视化成热力图。具体来说,将每一层的输出展开成网格,并将每个通道的特征图在网格上显示,同时对通道进行标准化和调整亮度,以便更好地观察特征图的细节。最后,将所有热力图显示在同一张图中,以便比较不同层之间的特征变化。
阅读全文
相关推荐
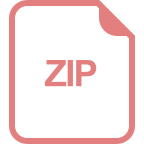


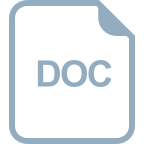
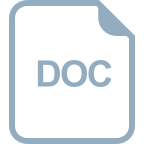
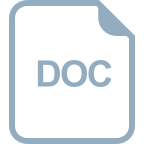










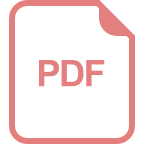