使用java程序来写
时间: 2023-12-14 11:40:04 浏览: 62
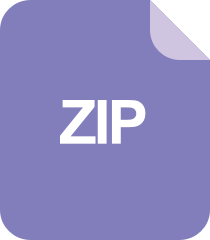
用Java写的一些程序
好的,使用Java编写类似ChatGPT的AI对话可以使用Java的自然语言处理库,例如Stanford CoreNLP或OpenNLP。以下是一个简单的示例代码:
```java
import java.util.*;
import edu.stanford.nlp.pipeline.*;
import edu.stanford.nlp.ling.*;
import edu.stanford.nlp.util.*;
public class Chatbot {
public static void main(String[] args) {
// set up pipeline
Properties props = new Properties();
props.setProperty("annotators", "tokenize, ssplit, pos, lemma, ner, parse, sentiment");
StanfordCoreNLP pipeline = new StanfordCoreNLP(props);
// start conversation
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("You: ");
String text = scanner.nextLine();
if (text.equalsIgnoreCase("exit")) {
System.out.println("Goodbye!");
break;
}
Annotation document = new Annotation(text);
pipeline.annotate(document);
List<CoreMap> sentences = document.get(CoreAnnotations.SentencesAnnotation.class);
for (CoreMap sentence : sentences) {
String sentiment = sentence.get(SentimentCoreAnnotations.SentimentClass.class);
System.out.println("Chatbot: " + generateResponse(sentiment));
}
}
scanner.close();
}
private static String generateResponse(String sentiment) {
// generate response based on sentiment
if (sentiment.equals("Positive")) {
return "That's great to hear!";
} else if (sentiment.equals("Negative")) {
return "I'm sorry to hear that.";
} else {
return "Hmm, I'm not sure what to say.";
}
}
}
```
此代码使用Stanford CoreNLP库来进行自然语言处理,将输入文本进行分析并提取情感。然后,根据情感生成对应的回复。在此示例中,Chatbot只是简单地根据情感生成回复,但您可以根据需要自定义生成回复的逻辑。
阅读全文
相关推荐
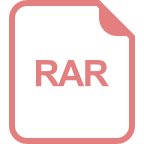
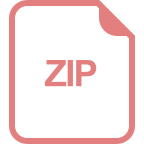
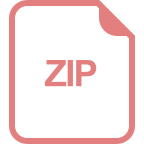
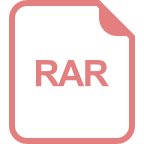
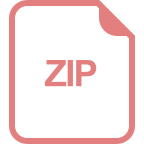
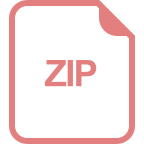
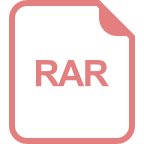
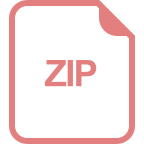
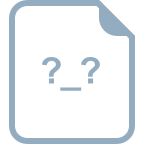