使用 ViewPager 和 Fragment 做一个简单版的好友列表界面 1. 使用 ViewPager 和 Fragment 做个可滑动界面 2. 使用 TabLayout 添加 Tab 支持给出文件的所有代码,包括import,以及配置。要求可以直接运行
时间: 2024-02-25 09:56:12 浏览: 183
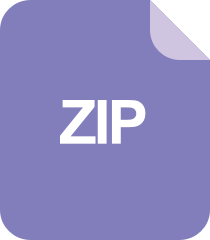
viewpager2 tablayout fragment, tab自定义
好的,以下是可滑动的好友列表界面的实现代码。
首先,我们需要创建一个包含 ViewPager 和 TabLayout 的布局文件,例如 `activity_main.xml`:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorPrimary"
android:minHeight="?attr/actionBarSize"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:tabGravity="fill"
app:tabIndicatorColor="@color/colorAccent"
app:tabMode="scrollable" />
<android.support.v4.view.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/tab_layout" />
</RelativeLayout>
```
接下来,我们需要创建两个 Fragment 类来展示好友列表,例如 `RecentFriendsFragment` 和 `AllFriendsFragment`。
`RecentFriendsFragment` 类的代码如下:
```java
public class RecentFriendsFragment extends Fragment {
public RecentFriendsFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_recent_friends, container, false);
}
}
```
`AllFriendsFragment` 类的代码和 `RecentFriendsFragment` 类的代码类似,这里不再赘述。
接下来,我们需要创建一个 Adapter 类来将两个 Fragment 添加到 ViewPager 中,例如 `FriendsPagerAdapter` 类:
```java
public class FriendsPagerAdapter extends FragmentPagerAdapter {
private String[] tabTitles = new String[]{"Recent", "All"};
public FriendsPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
switch (position) {
case 0:
return new RecentFriendsFragment();
case 1:
return new AllFriendsFragment();
default:
return null;
}
}
@Override
public int getCount() {
return tabTitles.length;
}
@Override
public CharSequence getPageTitle(int position) {
return tabTitles[position];
}
}
```
最后,在 `MainActivity` 中设置 ViewPager 和 TabLayout,例如:
```java
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
private TabLayout tabLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.view_pager);
tabLayout = findViewById(R.id.tab_layout);
FriendsPagerAdapter adapter = new FriendsPagerAdapter(getSupportFragmentManager());
viewPager.setAdapter(adapter);
tabLayout.setupWithViewPager(viewPager);
}
}
```
好了,以上就是使用 ViewPager 和 Fragment 做一个简单版的好友列表界面的全部代码。如果你需要详细的 import 和配置,可以参考下面的完整代码:
`activity_main.xml`:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorPrimary"
android:minHeight="?attr/actionBarSize"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:tabGravity="fill"
app:tabIndicatorColor="@color/colorAccent"
app:tabMode="scrollable" />
<android.support.v4.view.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/tab_layout" />
</RelativeLayout>
```
`fragment_recent_friends.xml` 和 `fragment_all_friends.xml`:
```xml
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="24sp" />
```
`RecentFriendsFragment` 和 `AllFriendsFragment`:
```java
public class RecentFriendsFragment extends Fragment {
public RecentFriendsFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_recent_friends, container, false);
}
}
public class AllFriendsFragment extends Fragment {
public AllFriendsFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_all_friends, container, false);
}
}
```
`FriendsPagerAdapter`:
```java
public class FriendsPagerAdapter extends FragmentPagerAdapter {
private String[] tabTitles = new String[]{"Recent", "All"};
public FriendsPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public Fragment getItem(int position) {
switch (position) {
case 0:
return new RecentFriendsFragment();
case 1:
return new AllFriendsFragment();
default:
return null;
}
}
@Override
public int getCount() {
return tabTitles.length;
}
@Override
public CharSequence getPageTitle(int position) {
return tabTitles[position];
}
}
```
`MainActivity`:
```java
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
private TabLayout tabLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.view_pager);
tabLayout = findViewById(R.id.tab_layout);
FriendsPagerAdapter adapter = new FriendsPagerAdapter(getSupportFragmentManager());
viewPager.setAdapter(adapter);
tabLayout.setupWithViewPager(viewPager);
}
}
```
注意需要在 `build.gradle` 中添加以下依赖:
```groovy
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support:design:28.0.0'
```
阅读全文
相关推荐
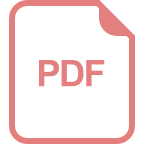
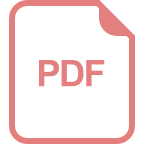














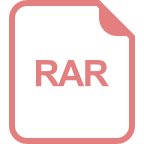
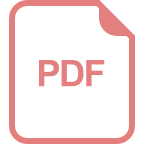