winform处理{ "msg": "success", "code": 0, "data": [ { "entName": "江苏快达农化股份有限公司总部", "type": "废水", "pointName": "快达(二期)", "monitorName": "流量", "monitorData": 0, "unit": "L/S", "monitorTime": "2023-05-31 21:13:10" }, { "entName": "江苏快达农化股份有限公司总部", "type": "废水", "pointName": "快达(二期)", "monitorName": "pH值", "monitorData": 8.05, "unit": null, "monitorTime": "2023-05-31 21:13:10" }, { "entName": "江苏快达农化股份有限公司总部", "type": "废水", "pointName": "快达(二期)", "monitorName": "COD", "monitorData": 195.4, "unit": "mg/L", "monitorTime": "2023-05-31 21:13:10" } ] }并找到"type":"废水"且"pointName":"快达(二期)"且"monitorName":"COD"的"monitorData"的值并输出
时间: 2023-07-03 21:24:50 浏览: 145
可以通过以下代码实现:
```csharp
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Threading.Tasks;
namespace WinFormDemo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private async void button1_Click(object sender, EventArgs e)
{
// 构造请求参数
string url = "http://example.com/api/data";
var parameters = new Dictionary<string, string>
{
{ "key", "value" }
};
// 发送 HTTP 请求
using (var client = new HttpClient())
{
var response = await client.PostAsync(url, new FormUrlEncodedContent(parameters));
if (response.IsSuccessStatusCode)
{
// 解析响应数据
var json = await response.Content.ReadAsStringAsync();
var result = JsonConvert.DeserializeObject<ApiResponse>(json);
// 遍历结果,找到符合条件的数据
foreach (var item in result.Data)
{
if (item.Type == "废水" && item.PointName == "快达(二期)" && item.MonitorName == "COD")
{
Console.WriteLine(item.MonitorData);
}
}
}
}
}
}
public class ApiResponse
{
public string Msg { get; set; }
public int Code { get; set; }
public List<DataItem> Data { get; set; }
}
public class DataItem
{
public string EntName { get; set; }
public string Type { get; set; }
public string PointName { get; set; }
public string MonitorName { get; set; }
public double MonitorData { get; set; }
public string Unit { get; set; }
public string MonitorTime { get; set; }
}
}
```
其中,ApiResponse 类和 DataItem 类用于解析响应数据。在 button1_Click 方法中,首先构造了一个 POST 请求,然后发送 HTTP 请求并解析响应数据。最后遍历结果,找到符合条件的数据并输出。
阅读全文
相关推荐
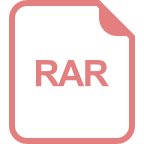
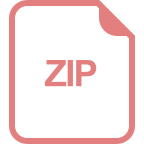
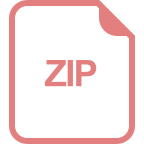
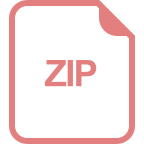
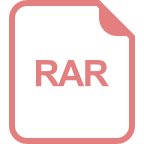
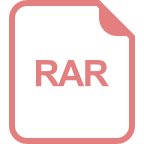
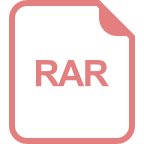
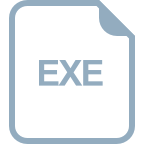
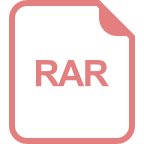
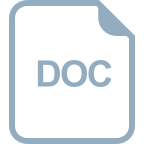
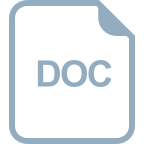
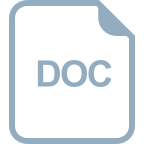
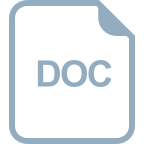
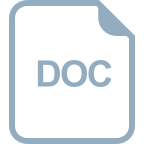
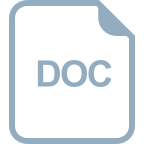
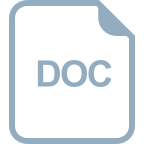
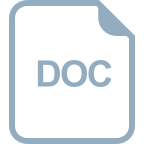