把这段代码加上注释,并详细一点
时间: 2024-03-11 12:49:40 浏览: 29
好的,以下是加上注释的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define CACHE_SIZE 5
// 定义结构体
typedef struct {
int id;
char name[30];
} Data;
typedef struct {
Data* buffer[CACHE_SIZE]; // 缓存数组
int front; // 缓存队列的头指针
int rear; // 缓存队列的尾指针
int count; // 缓存队列中元素的个数
} Cache;
// 初始化缓存
void initCache(Cache* cache) {
cache->front = 0;
cache->rear = CACHE_SIZE - 1;
cache->count = 0;
}
// 添加数据到缓存
void addToCache(Cache* cache, Data* data) {
// 如果缓存已满,则删除最老的数据
if (cache->count >= CACHE_SIZE) {
printf("Cache is full, removing oldest entry.\n");
free(cache->buffer[cache->front]); // 释放最老的数据
cache->front = (cache->front + 1) % CACHE_SIZE; // 更新头指针
cache->count--;
}
// 将数据添加到缓存队列的尾部
cache->rear = (cache->rear + 1) % CACHE_SIZE;
cache->buffer[cache->rear] = data;
cache->count++;
}
// 打印缓存中的所有数据
void printCache(Cache* cache) {
printf("Cache contents:\n");
int i, j;
for (i = 0, j = cache->front; i < cache->count; i++) {
printf("%d %s\n", cache->buffer[j]->id, cache->buffer[j]->name);
j = (j + 1) % CACHE_SIZE;
}
}
int main() {
Cache cache;
initCache(&cache); // 初始化缓存
// 向缓存中添加三条数据
Data* data1 = (Data*) malloc(sizeof(Data));
data1->id = 1;
sprintf(data1->name, "Data %d", data1->id);
addToCache(&cache, data1);
Data* data2 = (Data*) malloc(sizeof(Data));
data2->id = 2;
sprintf(data2->name, "Data %d", data2->id);
addToCache(&cache, data2);
Data* data3 = (Data*) malloc(sizeof(Data));
data3->id = 3;
sprintf(data3->name, "Data %d", data3->id);
addToCache(&cache, data3);
printCache(&cache); // 打印缓存中的所有数据
// 向缓存中添加一条数据,此时缓存已经满了,需要删除最老的数据
Data* data4 = (Data*) malloc(sizeof(Data));
data4->id = 4;
sprintf(data4->name, "Data %d", data4->id);
addToCache(&cache, data4);
printCache(&cache); // 打印缓存中的所有数据
// 向缓存中添加一条数据,此时缓存已经满了,需要删除最老的数据
Data* data5 = (Data*) malloc(sizeof(Data));
data5->id = 5;
sprintf(data5->name, "Data %d", data5->id);
addToCache(&cache, data5);
printCache(&cache); // 打印缓存中的所有数据
// 向缓存中添加一条数据,此时缓存已经满了,需要删除最老的数据
Data* data6 = (Data*) malloc(sizeof(Data));
data6->id = 6;
sprintf(data6->name, "Data %d", data6->id);
addToCache(&cache, data6);
printCache(&cache); // 打印缓存中的所有数据
return 0;
}
```
在这段代码中,首先定义了一个Data结构体用于存储数据,以及一个Cache结构体用于存储缓存。然后定义了三个函数:initCache函数用于初始化缓存,addToCache函数用于向缓存中添加数据,printCache函数用于打印缓存中的所有数据。最后在main函数中演示了如何使用这些函数。
具体而言,initCache函数将缓存队列的头指针设置为0,尾指针设置为缓存大小减1,元素个数设置为0。addToCache函数向缓存中添加数据,如果缓存已经满了,则删除最老的数据。printCache函数打印缓存中的所有数据。在main函数中,先通过initCache函数初始化缓存,然后向缓存中添加三条数据,并且打印缓存中的所有数据。接着向缓存中添加一条数据,此时缓存已经满了,需要删除最老的数据,并且再次打印缓存中的所有数据。最后再向缓存中添加两条数据,也需要删除最老的数据,并且打印缓存中的所有数据。
相关推荐
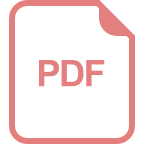
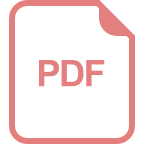
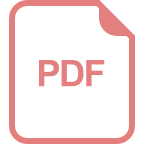














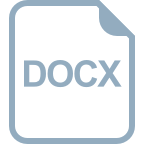