jsp连接数据库的步骤
时间: 2024-01-27 18:44:33 浏览: 20
连接数据库的步骤如下:
1. 导入数据库驱动包:在项目中导入 MySQL 或其他数据库的驱动包,以便能够使用 JDBC API 连接数据库。
2. 注册 JDBC 驱动程序:使用 Class.forName() 方法来加载驱动程序。例如,如果要连接 MySQL 数据库,则需要加载 com.mysql.jdbc.Driver 驱动程序。
3. 创建数据库连接:使用 DriverManager.getConnection() 方法创建与数据库的连接。需要提供数据库的 URL、用户名和密码。
4. 创建 Statement 对象:使用 Connection 对象的 createStatement() 方法创建 Statement 对象,从而可以向数据库发送 SQL 语句。
5. 执行 SQL 语句:使用 Statement 对象的 executeQuery() 或 executeUpdate() 方法执行 SQL 语句。
6. 处理查询结果:如果执行的 SQL 语句为查询语句,则需要使用 ResultSet 对象处理查询结果。
7. 关闭连接:在完成对数据库的操作后,需要关闭 Connection、Statement 和 ResultSet 对象。可以使用 try-with-resources 语句来自动关闭这些对象。
下面是一个示例代码:
```
import java.sql.*;
public class JSPJDBCExample {
public static void main(String args[]) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 导入 MySQL 驱动包
Class.forName("com.mysql.jdbc.Driver");
// 注册 JDBC 驱动程序并建立连接
String url = "jdbc:mysql://localhost/mydatabase";
String user = "root";
String password = "mypassword";
conn = DriverManager.getConnection(url, user, password);
// 创建 Statement 对象并执行 SQL 语句
stmt = conn.createStatement();
String sql = "SELECT id, name, age FROM employees";
rs = stmt.executeQuery(sql);
// 处理查询结果集
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭连接、Statement 和 ResultSet 对象
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
相关推荐
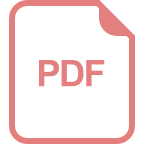
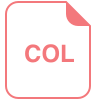
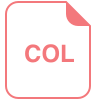












