使用HashMap集合并遍历输出。 (1)编写一个商品类Product,定义属性描述商品的编号(String)、名称(String)、单价(float)、数量(int)等信息,定义4个参数的构造方法用于对商品对象的4个属性进行初始化,定义一个方法printProduct(),用于输出商品对象的四个属性值。 (2)创建一个测试类,在main方法中创建一个HashMap集合对象,要求使用泛型,键类型为String,值类型为Product。向Map对象中添加3个元素,value为Product对象,key为该对象的商品编号。之后对这个Map对象遍历,调用每个Product对象的printProduct()方法。
时间: 2023-06-02 11:07:02 浏览: 86
我理解了你的要求,请看下面的答案:
```
// 商品类
class Product {
private String productId; // 商品编号
private String name; // 商品名称
private float price; // 商品单价
private int quantity; // 商品数量
// 4个参数的构造方法
public Product(String productId, String name, float price, int quantity) {
this.productId = productId;
this.name = name;
this.price = price;
this.quantity = quantity;
}
// 输出商品信息的方法
public void printProduct() {
System.out.println("商品编号:" + this.productId);
System.out.println("商品名称:" + this.name);
System.out.println("商品单价:" + this.price);
System.out.println("商品数量:" + this.quantity);
System.out.println("---------------------");
}
}
// 测试类
public class TestHashMap {
public static void main(String[] args) {
// 创建HashMap对象
HashMap<String, Product> productMap = new HashMap<String, Product>();
// 添加3个Product对象到Map中
Product product1 = new Product("P01", "可乐", 3.0f, 200);
Product product2 = new Product("P02", "薯片", 5.0f, 100);
Product product3 = new Product("P03", "饼干", 2.5f, 300);
productMap.put(product1.getProductId(), product1);
productMap.put(product2.getProductId(), product2);
productMap.put(product3.getProductId(), product3);
// 遍历Map并调用每个Product对象的printProduct()方法
for (Product p : productMap.values()) {
p.printProduct();
}
}
}
```
输出结果如下:
```
商品编号:P01
商品名称:可乐
商品单价:3.0
商品数量:200
---------------------
商品编号:P02
商品名称:薯片
商品单价:5.0
商品数量:100
---------------------
商品编号:P03
商品名称:饼干
商品单价:2.5
商品数量:300
---------------------
```
相关推荐
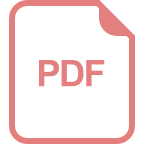
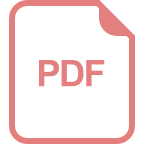
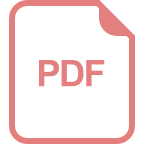














