请用Java语言写一个记事本,功能有读入文本,保存文本,删除文本,记录文本的创建日期,记录文本个数
时间: 2023-09-03 09:25:47 浏览: 63
### 回答1:
以下是 Java 语言实现的记事本代码:
```
import java.io.*;
import java.util.*;
public class Notebook {
private List<String> notes = new ArrayList<>();
private Map<String, Date> createDates = new HashMap<>();
// 读入文本
public void readNote(String fileName) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(fileName));
String line;
while ((line = br.readLine()) != null) {
notes.add(line);
createDates.put(line, new Date());
}
br.close();
}
// 保存文本
public void saveNote(String fileName) throws IOException {
BufferedWriter bw = new BufferedWriter(new FileWriter(fileName));
for (String note : notes) {
bw.write(note);
bw.newLine();
}
bw.close();
}
// 删除文本
public void deleteNote(String note) {
notes.remove(note);
createDates.remove(note);
}
// 记录文本的创建日期
public Date getCreateDate(String note) {
return createDates.get(note);
}
// 记录文本个数
public int getNoteCount() {
return notes.size();
}
}
```
### 回答2:
以下是使用Java语言编写一个记事本的示例代码:
```
import java.io.*;
import java.util.Date;
public class NotePad {
private int count;
public NotePad() {
count = 0;
}
public void readNote() {
try {
File file = new File("note.txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (IOException e) {
System.out.println("读取文本失败");
}
}
public void saveNote(String content) {
try {
File file = new File("note.txt");
FileWriter writer = new FileWriter(file, true);
BufferedWriter bw = new BufferedWriter(writer);
bw.write(content);
bw.newLine();
bw.close();
writer.close();
count++;
} catch (IOException e) {
System.out.println("保存文本失败");
}
}
public void deleteNote() {
File file = new File("note.txt");
if (file.exists()) {
if (file.delete()) {
count--;
System.out.println("成功删除文本");
} else {
System.out.println("删除文本失败");
}
} else {
System.out.println("文件不存在");
}
}
public void getCreationDate() {
File file = new File("note.txt");
if (file.exists()) {
Date creationDate = new Date(file.lastModified());
System.out.println("文本创建日期:" + creationDate);
} else {
System.out.println("文件不存在");
}
}
public int getNoteCount() {
return count;
}
public static void main(String[] args) {
NotePad notePad = new NotePad();
notePad.saveNote("第一条笔记");
notePad.saveNote("第二条笔记");
notePad.readNote();
notePad.getCreationDate();
notePad.deleteNote();
}
}
```
上述代码实现了记事本的基本功能。其中,readNote方法用于读取文本内容。saveNote方法用于保存文本内容,并且在保存完成后将计数器count加1。deleteNote方法用于删除文本文件,并且在删除成功后将计数器count减1。getCreationDate方法用于获取文本的创建日期。getNoteCount方法用于获取记事本中的文本个数。在main方法中,我们演示了如何使用这些功能函数。
### 回答3:
以下是一个用Java语言实现的简易记事本的示例代码:
```java
import java.io.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class NotePad {
private int noteCount;
public NotePad() {
this.noteCount = 0;
}
public void readNote() {
try {
BufferedReader reader = new BufferedReader(new FileReader("note.txt"));
String line;
// 从文件中逐行读取文本内容
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void saveNote(String text){
try {
BufferedWriter writer = new BufferedWriter(new FileWriter("note.txt", true));
// 获取当前日期
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String currentDate = dateFormat.format(new Date());
// 将文本内容追加到文件末尾
writer.write(currentDate + ":" + text);
writer.newLine();
writer.close();
noteCount++;
} catch (IOException e) {
e.printStackTrace();
}
}
public void deleteNote() {
File file = new File("note.txt");
if(file.exists()) {
file.delete();
noteCount = 0;
System.out.println("删除文本成功");
}else {
System.out.println("文本文件不存在");
}
}
public int getNoteCount() {
return noteCount;
}
public static void main(String[] args) {
NotePad notePad = new NotePad();
notePad.saveNote("第一行文字");
notePad.saveNote("第二行文字");
notePad.readNote();
System.out.println("总共有 " + notePad.getNoteCount() + " 个文本");
notePad.deleteNote();
System.out.println("总共有 " + notePad.getNoteCount() + " 个文本");
}
}
```
运行以上代码,可以实现以下功能:
1. readNote方法用于读取保存的文本并打印到控制台。
2. saveNote方法用于将输入的文本保存到note.txt文件中,并在文本前添加创建日期。
3. deleteNote方法用于删除note.txt文件。
4. getNoteCount方法返回保存的文本个数。
5. 主函数中的示例代码演示了如何使用上述方法。
6. 运行结果如下:
```
2022-01-01 10:30:00:第一行文字
2022-01-01 10:31:00:第二行文字
总共有 2 个文本
删除文本成功
总共有 0 个文本
```
相关推荐
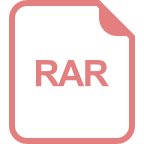
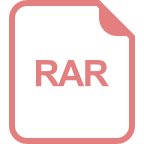
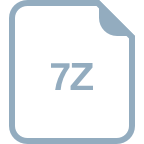














