利用Java Swing 图形组件开发一个图形化简易记事本。记事本功能包括文本编辑、保存文本到指定路径、打开指定路径下的文本、退出等
时间: 2023-11-29 15:07:14 浏览: 89
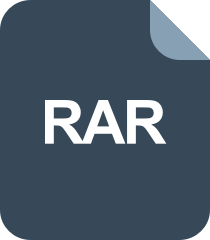
基于Java+Swing实现记事本-完美版

好的,我可以为您提供一个简单的Java Swing记事本示例代码,包括文本编辑、保存和打开文件、退出等功能。请参考以下代码:
```
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class SimpleNotepad extends JFrame implements ActionListener {
private JTextArea textArea;
private JFileChooser fileChooser;
private File file;
private static final long serialVersionUID = 1L;
public SimpleNotepad() {
setTitle("Simple Notepad");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 600);
// Create the menu bar and add the menus
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem newMenuItem = new JMenuItem("New");
newMenuItem.addActionListener(this);
JMenuItem openMenuItem = new JMenuItem("Open");
openMenuItem.addActionListener(this);
JMenuItem saveMenuItem = new JMenuItem("Save");
saveMenuItem.addActionListener(this);
JMenuItem exitMenuItem = new JMenuItem("Exit");
exitMenuItem.addActionListener(this);
fileMenu.add(newMenuItem);
fileMenu.add(openMenuItem);
fileMenu.add(saveMenuItem);
fileMenu.addSeparator();
fileMenu.add(exitMenuItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// Create the text area
textArea = new JTextArea(30, 60);
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// Create the button panel
JPanel buttonPanel = new JPanel();
JButton saveButton = new JButton("Save");
saveButton.addActionListener(this);
JButton openButton = new JButton("Open");
openButton.addActionListener(this);
buttonPanel.add(saveButton);
buttonPanel.add(openButton);
add(buttonPanel, BorderLayout.SOUTH);
// Create the file chooser
fileChooser = new JFileChooser();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("New")) {
textArea.setText("");
file = null;
} else if (e.getActionCommand().equals("Open")) {
int returnVal = fileChooser.showOpenDialog(SimpleNotepad.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
try {
file = fileChooser.getSelectedFile();
BufferedReader reader = new BufferedReader(new FileReader(file));
String line = null;
textArea.setText("");
while ((line = reader.readLine()) != null) {
textArea.append(line + "\n");
}
reader.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
} else if (e.getActionCommand().equals("Save")) {
if (file == null) {
int returnVal = fileChooser.showSaveDialog(SimpleNotepad.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
file = fileChooser.getSelectedFile();
}
}
if (file != null) {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(textArea.getText());
writer.close();
} catch (Exception ex) {
ex.printStackTrace();
}
}
} else if (e.getActionCommand().equals("Exit")) {
System.exit(0);
}
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
new SimpleNotepad();
}
});
}
}
```
该代码创建了一个带有文本区域和菜单栏的窗口,用户可以使用菜单栏中的选项打开、保存或退出文本文件。用户还可以使用文本区域编辑文本内容。在保存和打开文件时,该代码将使用文件选择器来允许用户选择要保存或打开的文件。
阅读全文
相关推荐
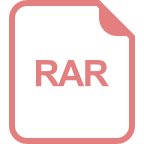

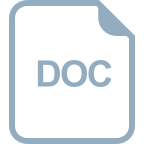
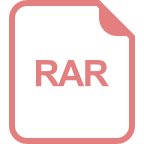
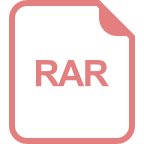
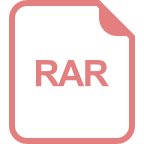
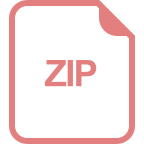
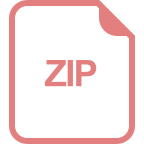
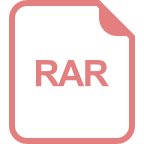
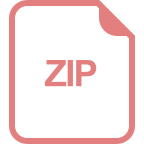
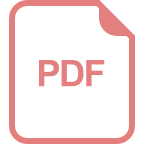
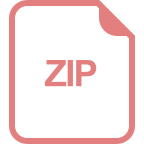
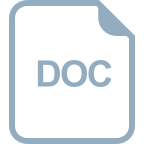
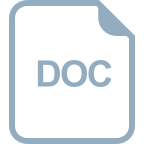
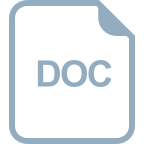
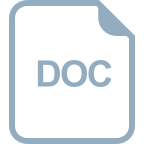
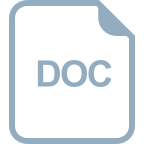