Java写题目1:电子元器件仓库管理系统 >应有一个友好,逻辑清晰的软件界面 >电子元器件的入库、出库 >电子元器件的属性标识 >具备元器件库存不足报警功能 > 具备按照不同类型查看相关元器件的功能 >具有一定的统计功能,如在库元器件总价值、每月出库元器件总价值、各类元器件的月(周)消耗量等
时间: 2024-01-29 13:13:22 浏览: 72
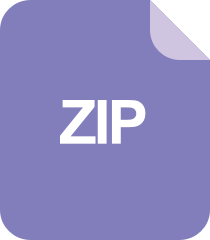
题目:电子元器件仓库管理系统
以下是一个简单的 Java 实现,实现了上述需求:
1. 界面设计:
使用 JavaFX 框架,界面包含表格来显示库存信息,使用按钮和文本框来实现入库、出库等操作。下拉框用来选择不同类型的元器件。
2. 元器件的入库、出库:
使用内存数据结构来存储库存信息,当按钮被点击时先进行输入合法性检查,然后进行入库或出库操作。在入库操作中,需要添加元器件的属性标识。在出库操作中,需要判断库存是否充足,若不充足,则发出报警提示。
3. 元器件的属性标识:
创建一个元器件类,包含元器件的各种属性,如名称、类型、单价、数量等。在入库操作中,创建元器件对象并添加到库存中,同时在界面上显示元器件的属性信息。在出库操作中,需要根据元器件的属性来判断是否符合要求。
4. 库存不足报警功能:
在出库操作中添加判断库存是否充足的逻辑,若库存不足,则发出报警提示。
5. 按照不同类型查看相关元器件的功能:
使用下拉框让用户选择不同类型的元器件,选择不同的选项后,显示相应类型的元器件信息。
6. 统计功能:
在界面上添加一个按钮,点击后弹出统计信息的窗口。统计信息包括在库元器件总价值、每月出库元器件总价值、各类元器件的月(周)消耗量等。使用 Java 时间类来处理日期数据,使用 Java 集合类来管理库存。在统计信息中,需要对库存中的元器件进行遍历和计算。
下面是代码实现的一些简单示例:
```
public class ElectronicComponent {
private String name;
private String type;
private double price;
private int quantity;
public ElectronicComponent(String name, String type, double price, int quantity) {
this.name = name;
this.type = type;
this.price = price;
this.quantity = quantity;
}
// 省略 getter 和 setter 方法
}
public class Inventory {
private List<ElectronicComponent> components;
public Inventory() {
components = new ArrayList<>();
}
public void addComponent(ElectronicComponent component) {
components.add(component);
}
public void removeComponent(ElectronicComponent component) {
components.remove(component);
}
// 统计库存总价值
public double getTotalValue() {
double totalValue = 0.0;
for (ElectronicComponent component : components) {
totalValue += component.getPrice() * component.getQuantity();
}
return totalValue;
}
// 统计每月出库元器件总价值
public double getMonthlyOutValue(int year, int month) {
double monthlyOutValue = 0.0;
for (ElectronicComponent component : components) {
// 省略判断该元器件是否在该月有出库记录的逻辑
monthlyOutValue += component.getPrice() * component.getQuantity();
}
return monthlyOutValue;
}
// 统计某种类型元器件的消耗量
public int getComponentConsumption(String type, int year, int month) {
int consumption = 0;
for (ElectronicComponent component : components) {
if (component.getType().equals(type)) {
// 省略判断该元器件在该月是否出库的逻辑
consumption += component.getQuantity();
}
}
return consumption;
}
// 根据元器件类型过滤库存信息
public List<ElectronicComponent> filterInventory(String type) {
List<ElectronicComponent> filteredComponents = new ArrayList<>();
for (ElectronicComponent component : components) {
if (component.getType().equals(type)) {
filteredComponents.add(component);
}
}
return filteredComponents;
}
// 省略其他方法
}
public class Main extends Application {
private Inventory inventory;
private TableView<ElectronicComponent> componentTable;
private TextField nameField;
private ComboBox<String> typeBox;
private TextField priceField;
private TextField quantityField;
private Button addButton;
private Button removeButton;
private Button outButton;
private Label messageLabel;
@Override
public void start(Stage primaryStage) throws Exception {
// 界面布局
componentTable = new TableView<>();
nameField = new TextField();
typeBox = new ComboBox<>();
priceField = new TextField();
quantityField = new TextField();
addButton = new Button("入库");
removeButton = new Button("删除");
outButton = new Button("出库");
messageLabel = new Label();
// 创建元器件类型下拉框
ObservableList<String> types = FXCollections.observableArrayList("电容器", "电阻器", "晶体管");
typeBox.setItems(types);
// 添加表格列
TableColumn<ElectronicComponent, String> nameColumn = new TableColumn<>("名称");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn<ElectronicComponent, String> typeColumn = new TableColumn<>("类型");
typeColumn.setCellValueFactory(new PropertyValueFactory<>("type"));
TableColumn<ElectronicComponent, Double> priceColumn = new TableColumn<>("单价");
priceColumn.setCellValueFactory(new PropertyValueFactory<>("price"));
TableColumn<ElectronicComponent, Integer> quantityColumn = new TableColumn<>("数量");
quantityColumn.setCellValueFactory(new PropertyValueFactory<>("quantity"));
componentTable.getColumns().addAll(nameColumn, typeColumn, priceColumn, quantityColumn);
// 添加事件监听器
addButton.setOnAction(event -> addComponent());
removeButton.setOnAction(event -> removeComponent());
outButton.setOnAction(event -> outComponent());
// 界面布局代码省略
// 初始化库存
inventory = new Inventory();
inventory.addComponent(new ElectronicComponent("电容1", "电容器", 0.5, 100));
inventory.addComponent(new ElectronicComponent("电容2", "电容器", 0.8, 200));
inventory.addComponent(new ElectronicComponent("电阻1", "电阻器", 0.2, 500));
inventory.addComponent(new ElectronicComponent("电阻2", "电阻器", 0.3, 600));
inventory.addComponent(new ElectronicComponent("晶体管1", "晶体管", 1.2, 50));
// 更新表格中的数据
componentTable.setItems(FXCollections.observableArrayList(inventory.getComponents()));
}
private void addComponent() {
String name = nameField.getText();
String type = typeBox.getValue();
double price = Double.parseDouble(priceField.getText());
int quantity = Integer.parseInt(quantityField.getText());
// 省略输入合法性检查
ElectronicComponent component = new ElectronicComponent(name, type, price, quantity);
inventory.addComponent(component);
componentTable.getItems().add(component);
}
private void removeComponent() {
ElectronicComponent component = componentTable.getSelectionModel().getSelectedItem();
if (component != null) {
inventory.removeComponent(component);
componentTable.getItems().remove(component);
}
}
private void outComponent() {
ElectronicComponent component = componentTable.getSelectionModel().getSelectedItem();
if (component == null) {
messageLabel.setText("请选择元器件");
return;
}
int quantity = Integer.parseInt(quantityField.getText());
if (component.getQuantity() < quantity) {
messageLabel.setText("库存不足");
return;
}
// 省略出库逻辑
componentTable.refresh();
}
// 省略其他方法
}
```
这是一个简单的实现,具体的实现细节和界面设计可以根据需求进行修改。
阅读全文
相关推荐

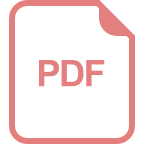
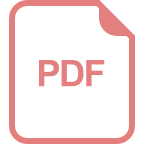
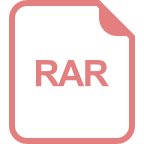
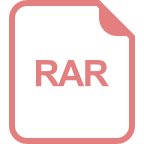
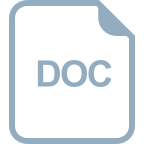
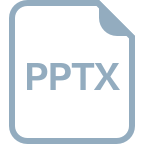
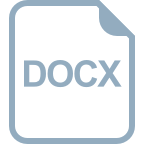
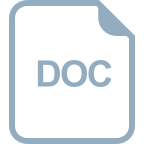
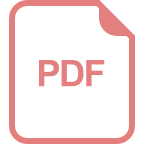
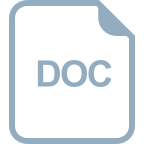
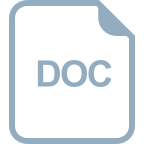
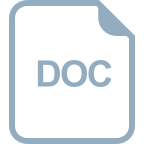

