vue 封装一个轮播图
时间: 2023-05-11 18:02:26 浏览: 89
可以使用 Vue 的组件化思想来封装一个轮播图。首先,需要创建一个 Carousel 组件,然后在该组件中使用 Vue 的生命周期函数来实现轮播图的自动播放和手动切换。具体实现可以参考以下代码:
<template>
<div class="carousel">
<div class="carousel-inner">
<div v-for="(item, index) in items" :key="index" :class="{ active: index === currentIndex }">
<img :src="item.src" alt="">
</div>
</div>
<a class="carousel-control-prev" href="#" role="button" @click="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#" role="button" @click="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
</template>
<script>
export default {
name: 'Carousel',
props: {
items: {
type: Array,
required: true
}
},
data() {
return {
currentIndex: 0,
timer: null
}
},
mounted() {
this.start()
},
methods: {
start() {
this.timer = setInterval(() => {
this.next()
}, 3000)
},
stop() {
clearInterval(this.timer)
this.timer = null
},
prev() {
this.currentIndex--
if (this.currentIndex < 0) {
this.currentIndex = this.items.length - 1
}
},
next() {
this.currentIndex++
if (this.currentIndex >= this.items.length) {
this.currentIndex = 0
}
}
},
watch: {
currentIndex() {
this.stop()
this.start()
}
}
}
</script>
<style>
.carousel {
position: relative;
width: 100%;
height: 300px;
overflow: hidden;
}
.carousel-inner {
position: absolute;
width: 100%;
height: 100%;
display: flex;
transition: transform 0.5s ease-in-out;
}
.carousel-inner > div {
flex: 1;
display: flex;
justify-content: center;
align-items: center;
}
.carousel-inner > div > img {
max-width: 100%;
max-height: 100%;
}
.carousel-inner > .active {
transform: translateX(0);
}
.carousel-inner > .prev {
transform: translateX(-100%);
}
.carousel-inner > .next {
transform: translateX(100%);
}
.carousel-control-prev,
.carousel-control-next {
position: absolute;
top: 50%;
transform: translateY(-50%);
z-index: 1;
display: flex;
justify-content: center;
align-items: center;
width: 50px;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
border-radius: 50%;
transition: background-color 0.2s ease-in-out;
}
.carousel-control-prev:hover,
.carousel-control-next:hover {
background-color: rgba(0, 0, 0, 0.8);
}
.carousel-control-prev-icon,
.carousel-control-next-icon {
display: inline-block;
width: 20px;
height: 20px;
background-repeat: no-repeat;
background-position: center;
background-size: contain;
fill: #fff;
}
.carousel-control-prev-icon {
background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 8 8'%3E%3Cpath d='M6.5 0l-3 3 3 3v-2h1v-2h-1v-2z'/%3E%3C/svg%3E");
}
.carousel-control-next-icon {
background-image: url("data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 8 8'%3E%3Cpath d='M1.5 0l3 3-3 3v-2h-1v-2h1v-2z'/%3E%3C/svg%3E");
}
</style>
在父组件中,可以通过以下方式来使用 Carousel 组件:
<template>
<div class="app">
<carousel :items="items"></carousel>
</div>
</template>
<script>
import Carousel from './Carousel.vue'
export default {
name: 'App',
components: {
Carousel
},
data() {
return {
items: [
{ src: 'https://picsum.photos/800/300?random=1' },
{ src: 'https://picsum.photos/800/300?random=2' },
{ src: 'https://picsum.photos/800/300?random=3' },
{ src: 'https://picsum.photos/800/300?random=4' },
{ src: 'https://picsum.photos/800/300?random=5' }
]
}
}
}
</script>
<style>
.app {
max-width: 800px;
margin: 0 auto;
}
</style>
这样就可以在页面上展示一个轮播图了。
相关推荐
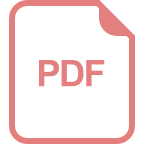














