用c语言写一段车牌识别代码
时间: 2023-10-15 13:06:58 浏览: 64
车牌识别技术需要使用图像处理和分析技术,由于涉及到很多复杂的算法和数据结构,因此需要使用专业的图像处理库来实现。下面是一个使用 OpenCV 库实现车牌识别的示例代码:
```c
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取输入图像
Mat src = imread(argv[1], IMREAD_COLOR);
if (src.empty())
{
cout << "Could not open or find the image!\n" << endl;
return -1;
}
// 转换为灰度图像
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 进行边缘检测
Mat edges;
Canny(gray, edges, 50, 150);
// 查找轮廓
vector<vector<Point>> contours;
findContours(edges, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 查找符合条件的矩形轮廓
vector<Rect> plates;
for (size_t i = 0; i < contours.size(); i++)
{
Rect rect = boundingRect(contours[i]);
float ratio = float(rect.width) / float(rect.height);
if (ratio > 2.0 && ratio < 6.0 && rect.width > 100 && rect.height > 30)
{
plates.push_back(rect);
}
}
// 绘制车牌区域
for (size_t i = 0; i < plates.size(); i++)
{
rectangle(src, plates[i], Scalar(0, 0, 255), 2);
}
// 显示结果图像
imshow("Plate Detection", src);
waitKey(0);
return 0;
}
```
以上代码实现了车牌区域的定位和绘制,具体实现原理请参考注释。需要注意的是,这只是一个简单的车牌识别示例,要实现完整的车牌识别功能,还需要使用更复杂的算法和技术,并且需要进行大量的优化和调试。
相关推荐
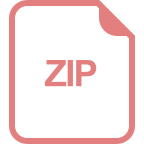
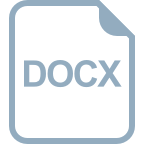














