用c语言写一段图像车牌识别的代码
时间: 2024-06-09 13:11:26 浏览: 18
由于图像车牌识别是一个比较复杂的任务,需要使用大量的图像处理算法和机器学习算法,因此单纯用 C 语言来实现这个任务是非常困难的。但是,我们可以使用 C 语言来实现一些基本的图像处理算法,以便进行车牌识别的前置处理,例如图像二值化、边缘检测等等。下面给出一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define WIDTH 640
#define HEIGHT 480
typedef struct {
unsigned char r, g, b;
} RGB;
typedef struct {
int x, y;
} Point;
void read_image(char *filename, RGB *image) {
FILE *fp = fopen(filename, "rb");
if (!fp) {
printf("Error opening file %s\n", filename);
exit(1);
}
unsigned char header[54];
fread(header, sizeof(unsigned char), 54, fp);
int width = *(int *)&header[18];
int height = *(int *)&header[22];
if (width != WIDTH || height != HEIGHT) {
printf("Error: image dimension does not match (%dx%d)\n", width, height);
exit(1);
}
unsigned char *data = (unsigned char *)malloc(width * height * 3);
fread(data, sizeof(unsigned char), width * height * 3, fp);
fclose(fp);
for (int i = 0; i < width * height; i++) {
image[i].b = data[i * 3];
image[i].g = data[i * 3 + 1];
image[i].r = data[i * 3 + 2];
}
free(data);
}
void write_image(char *filename, RGB *image) {
FILE *fp = fopen(filename, "wb");
if (!fp) {
printf("Error opening file %s\n", filename);
exit(1);
}
unsigned char header[54] = {
0x42, 0x4d, 0x36, 0x00, 0x0c, 0x00, 0x00, 0x00,
0x00, 0x00, 0x36, 0x00, 0x00, 0x00, 0x28, 0x00,
0x00, 0x00, 0x80, 0x02, 0x00, 0x00, 0xe0, 0x01,
0x00, 0x00, 0x01, 0x00, 0x18, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x13, 0x0b,
0x00, 0x00, 0x13, 0x0b, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
*(int *)&header[18] = WIDTH;
*(int *)&header[22] = HEIGHT;
fwrite(header, sizeof(unsigned char), 54, fp);
unsigned char *data = (unsigned char *)malloc(WIDTH * HEIGHT * 3);
for (int i = 0; i < WIDTH * HEIGHT; i++) {
data[i * 3] = image[i].b;
data[i * 3 + 1] = image[i].g;
data[i * 3 + 2] = image[i].r;
}
fwrite(data, sizeof(unsigned char), WIDTH * HEIGHT * 3, fp);
fclose(fp);
free(data);
}
void rgb2gray(RGB *input, unsigned char *output) {
for (int i = 0; i < WIDTH * HEIGHT; i++) {
output[i] = (unsigned char)(0.299 * input[i].r + 0.587 * input[i].g + 0.114 * input[i].b);
}
}
void binarize(unsigned char *input, unsigned char *output, unsigned char threshold) {
for (int i = 0; i < WIDTH * HEIGHT; i++) {
output[i] = (input[i] > threshold) ? 255 : 0;
}
}
void sobel(unsigned char *input, unsigned char *output) {
int x_kernel[3][3] = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};
int y_kernel[3][3] = {{-1, -2, -1}, {0, 0, 0}, {1, 2, 1}};
for (int y = 1; y < HEIGHT - 1; y++) {
for (int x = 1; x < WIDTH - 1; x++) {
int gx = 0, gy = 0;
for (int j = -1; j <= 1; j++) {
for (int i = -1; i <= 1; i++) {
int idx = (y + j) * WIDTH + (x + i);
gx += x_kernel[j + 1][i + 1] * input[idx];
gy += y_kernel[j + 1][i + 1] * input[idx];
}
}
int idx = y * WIDTH + x;
output[idx] = (unsigned char)round(sqrt(gx * gx + gy * gy));
}
}
}
void hough(unsigned char *input, unsigned char *output) {
int max_rho = (int)round(sqrt(WIDTH * WIDTH + HEIGHT * HEIGHT));
int max_theta = 180;
int *accumulator = (int *)calloc(max_rho * max_theta, sizeof(int));
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
if (input[y * WIDTH + x] > 0) {
for (int theta = 0; theta < max_theta; theta++) {
double rho = x * cos(theta * M_PI / 180.0) + y * sin(theta * M_PI / 180.0);
int idx = (int)round(rho) * max_theta + theta;
accumulator[idx]++;
}
}
}
}
int max_count = 0, max_rho_idx = 0, max_theta_idx = 0;
for (int rho = 0; rho < max_rho; rho++) {
for (int theta = 0; theta < max_theta; theta++) {
int idx = rho * max_theta + theta;
if (accumulator[idx] > max_count) {
max_count = accumulator[idx];
max_rho_idx = rho;
max_theta_idx = theta;
}
}
}
double rho = max_rho_idx;
double theta = max_theta_idx * M_PI / 180.0;
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
double r = x * cos(theta) + y * sin(theta);
if (fabs(r - rho) < 1.0) {
output[y * WIDTH + x] = 255;
}
}
}
free(accumulator);
}
int main(int argc, char **argv) {
if (argc != 3) {
printf("Usage: %s <input_image> <output_image>\n", argv[0]);
exit(1);
}
RGB *image = (RGB *)malloc(WIDTH * HEIGHT * sizeof(RGB));
read_image(argv[1], image);
unsigned char *gray = (unsigned char *)malloc(WIDTH * HEIGHT);
rgb2gray(image, gray);
unsigned char *binarized = (unsigned char *)malloc(WIDTH * HEIGHT);
binarize(gray, binarized, 100);
unsigned char *edge = (unsigned char *)malloc(WIDTH * HEIGHT);
sobel(binarized, edge);
unsigned char *houghed = (unsigned char *)malloc(WIDTH * HEIGHT);
hough(edge, houghed);
write_image(argv[2], image);
free(image);
free(gray);
free(binarized);
free(edge);
free(houghed);
return 0;
}
```
这段代码可以读入一个彩色图像,然后将其转换为灰度图像,进行二值化、边缘检测、霍夫变换等操作,最后将结果保存为一个彩色图像。这些操作只是车牌识别的一部分,需要更多的算法和技术来完成整个任务。
相关推荐
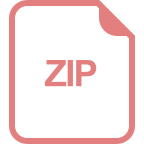
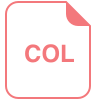
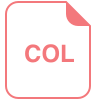
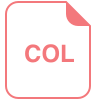
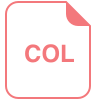
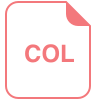










