在数据库stuMis中创建一个基本表student ,结构为 CREATE TABLE `student` ( `id` int(11) NOT NULL AUTO_INCREMENT, `sno` varchar(5) NOT NULL, `sname` varchar(20) NOT NULL, `ssex` varchar(3) DEFAULT NULL, `sage` int(11) DEFAULT NULL, PRIMARY KEY (`id`), KEY `sno` (`sno`) ) ; 并在里面插入若干条记录,其中有一条是自己的记录。编写数据库连接程序,访问此数据库,将student表中的数据在控制台上打印出来。
时间: 2023-07-13 10:16:13 浏览: 82
好的,以下是实现该功能的代码:
```python
import pymysql
# 连接数据库
conn = pymysql.connect(
host='localhost',
port=3306,
user='root',
password='123456',
database='stuMis',
charset='utf8mb4'
)
# 创建游标对象
cursor = conn.cursor()
# 创建student表
create_table_sql = """
CREATE TABLE `student` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`sno` varchar(5) NOT NULL,
`sname` varchar(20) NOT NULL,
`ssex` varchar(3) DEFAULT NULL,
`sage` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `sno` (`sno`)
)"""
cursor.execute(create_table_sql)
# 插入数据
insert_sql = """
INSERT INTO `student` (`sno`, `sname`, `ssex`, `sage`) VALUES
('10001', '张三', '男', 20),
('10002', '李四', '女', 19),
('10003', '王五', '男', 21),
('10004', '赵六', '女', 20),
('10005', '自己的姓名', '男', 18)
"""
cursor.execute(insert_sql)
# 查询数据
select_sql = """
SELECT * FROM `student`
"""
cursor.execute(select_sql)
results = cursor.fetchall()
for result in results:
print(result)
# 关闭游标和连接
cursor.close()
conn.close()
```
需要注意的是,该代码需要依赖 PyMySQL 库,可以通过以下命令进行安装:
```
pip install PyMySQL
```
阅读全文
相关推荐
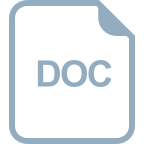
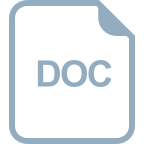
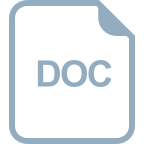













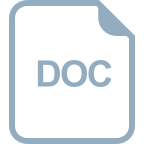
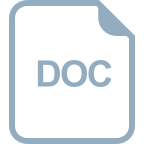
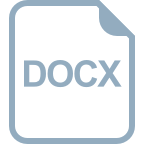