C++makefile文件中-R和-L有什么区别
时间: 2023-06-02 12:07:23 浏览: 137
-R 和 -L 都是 makefile 文件中链接选项,不过它们的作用是不同的。-L 用于指定编译器在链接文件时查找库文件的路径,通常用于指定库文件的目录。而 -R 则是让编译器在程序运行时动态链接共享库时,先在指定的路径中寻找库文件,如果找到,则使用该库文件。通常用于指定共享库文件的路径。
相关问题
Makefile文件里 -define的用处?和后面跟什么?
-define在Makefile中的作用是定义一个多行的变量,这个变量可以在整个Makefile中使用。-define后面跟着的是变量名,然后是变量的值,这个值可以跨越多行,直到一个以endef为关键字的行出现为止。例如:
```
-define VARIABLE_NAME
This is a multi-line variable
It can be used throughout the Makefile
endef
$(info $(VARIABLE_NAME))
```
在上面的例子中,-define定义了一个名为VARIABLE_NAME的多行变量,然后通过$(info)函数输出了这个变量的值。在Makefile中,可以使用$(VARIABLE_NAME)来引用这个变量。
需要注意的是,-define和endef之间不能有空格或其他字符,否则会导致错误。
在Makefile中,-l选项用于指定需要链接的库文件是静态链接还是动态链接
在Makefile中,-l选项用于指定需要链接的库文件,可以是静态库,也可以是动态库。
当链接静态库时,指定的库文件应该以".a"为后缀,例如:-lmylib(表示链接名为libmylib.a的静态库文件)。
当链接动态库时,指定的库文件应该以".so"为后缀,例如:-lmylib(表示链接名为libmylib.so的动态库文件)。
需要注意的是,如果同时存在同名的静态库和动态库,链接器会优先选择动态库进行链接。如果需要强制链接静态库,可以使用"-static"选项。
例如,以下语句用于链接名为libmylib.a的静态库文件:
```
LDFLAGS = -L/path/to/lib -lmylib
```
以下语句用于链接名为libmylib.so的动态库文件:
```
LDFLAGS = -L/path/to/lib -lmylib
```
以下语句用于强制链接名为libmylib.a的静态库文件:
```
LDFLAGS = -L/path/to/lib -static -lmylib
```
相关推荐
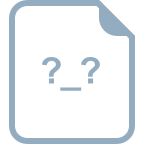
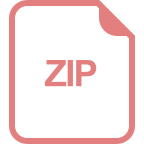
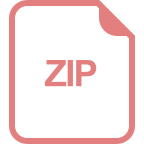












