请修改代码,模拟实现多个产品的放入与取出。
时间: 2024-04-30 21:25:12 浏览: 73
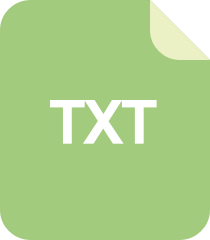
生产者消费者问题程序模拟实现,实用VC实现
好的,以下是一个简单的示例代码:
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
class Warehouse:
def __init__(self):
self.products = {}
def add_product(self, product, quantity):
if product.name in self.products:
self.products[product.name] += quantity
else:
self.products[product.name] = quantity
def remove_product(self, product, quantity):
if product.name in self.products:
if self.products[product.name] >= quantity:
self.products[product.name] -= quantity
return True
else:
return False
else:
return False
warehouse = Warehouse()
product1 = Product("iPhone", 999)
product2 = Product("iPad", 799)
product3 = Product("MacBook", 1299)
warehouse.add_product(product1, 10)
warehouse.add_product(product2, 5)
warehouse.add_product(product3, 2)
print(warehouse.products) # {'iPhone': 10, 'iPad': 5, 'MacBook': 2}
warehouse.remove_product(product1, 3)
warehouse.remove_product(product2, 2)
print(warehouse.products) # {'iPhone': 7, 'iPad': 3, 'MacBook': 2}
```
在这个示例代码中,我们定义了一个 `Product` 类来表示一个产品,其中包含名称和价格两个属性。我们还定义了一个 `Warehouse` 类来表示仓库,其中包含一个字典 `products`,用于存储不同产品的数量。
`Warehouse` 类中有两个方法,`add_product` 和 `remove_product`,分别用于添加和移除产品。这两个方法都接受一个 `Product` 对象和一个数量作为参数。在 `add_product` 方法中,我们检查该产品是否已经存在于仓库中,如果是,则将数量增加到已有数量上,否则将该产品添加到字典中。在 `remove_product` 方法中,我们首先检查该产品是否存在于仓库中,如果是,则检查仓库中是否有足够的产品数量可供移除,如果是,则将数量减去指定数量,否则返回 False。
最后,我们创建了三个 `Product` 对象,将它们添加到仓库中,并尝试移除一些产品,最终打印出仓库中的产品数量。
阅读全文
相关推荐
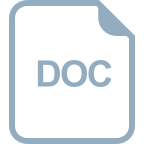
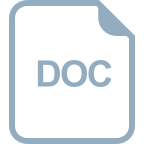











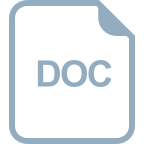
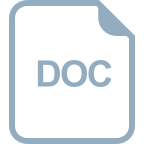
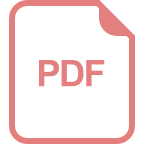
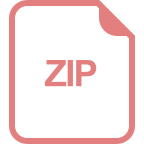
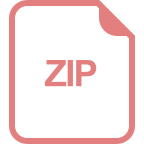
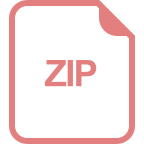