Java多线程写文件
时间: 2023-07-06 11:14:00 浏览: 82
在Java中,多线程写文件的实现与Python类似,也需要注意线程安全和写入效率的问题。下面是一个Java多线程写文件的示例代码:
```java
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterThread extends Thread {
private FileWriter writer;
private String data;
public FileWriterThread(FileWriter writer, String data) {
this.writer = writer;
this.data = data;
}
public void run() {
try {
writer.write(data);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) throws InterruptedException {
FileWriter writer = null;
try {
writer = new FileWriter("test.txt");
for (int i = 0; i < 10; i++) {
FileWriterThread thread = new FileWriterThread(writer, "thread " + i + "\n");
thread.start();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (writer != null) {
try {
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
在这个示例中,我们创建了一个 `FileWriterThread` 类来封装文件的写入操作,并在 `run` 方法中执行写入操作。然后创建了多个线程,每个线程都会调用 `FileWriterThread` 类的 `run` 方法来写入数据。最后,关闭文件。
需要注意的是,在Java中,如果多个线程同时写同一个文件,可能会出现数据错乱的情况。为了避免这种情况,可以使用锁机制来控制文件的访问,或者将文件分成多个部分,每个线程负责写入其中的一部分。
阅读全文
相关推荐











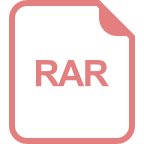

