result = mycursor.fetchone()[0]
时间: 2023-10-21 19:07:17 浏览: 27
这段代码是从 MySQL 数据库中获取查询结果集的第一行,并返回该行的第一个字段的值。更具体地说,`mycursor.fetchone()` 方法返回查询结果的第一行,然后 `[0]` 表示返回该行的第一个字段的值。
请注意,如果查询结果集为空,那么 `mycursor.fetchone()` 方法将返回 `None`,如果你尝试在 `None` 上执行 `[0]` 操作,会引发 `TypeError` 异常。因此,你应该在使用结果集之前检查它是否为空。例如:
```
result = mycursor.fetchone()
if result is not None:
# 对结果集进行操作
value = result[0]
else:
# 处理结果集为空的情况
value = None
```
相关问题
cursor.fetchone
`cursor.fetchone()`是Python中用于从数据库中获取一条记录的方法。它可以在使用Python连接数据库时,通过游标对象来获取查询结果集中的一条记录。该方法会返回一个包含该记录数据的元组或者None(如果没有更多的记录)。使用该方法时,需要先使用`cursor.execute()`方法执行SQL查询语句,然后再使用`cursor.fetchone()`方法获取查询结果集中的一条记录。
例如,以下代码演示了如何使用`cursor.fetchone()`方法从数据库中获取一条记录:
```
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行SQL查询语句
mycursor.execute("SELECT * FROM customers")
# 获取查询结果集中的第一条记录
result = mycursor.fetchone()
# 输出该记录
print(result)
```
k.Label(login_frame, text='账号:',bg='white').grid(row=0, column=0,pady=10) tk.Entry(login_frame,bd=2).grid(row=0, column=1,pady=10) tk.Label(login_frame, text='密码:',bg='white').grid(row=1, column=0,pady=10) tk.Entry(login_frame, show='*',bd=2).grid(row=1, column=1,pady=10)优化这段代码,得到输入框输入得数据与MySQL里表里面得数据进行对比python
可以将输入框的数据存储到变量中,然后在点击登录按钮时,使用Python的MySQL模块连接到MySQL数据库,查询表中是否存在该账号密码对应的记录,如果存在则登录成功,否则登录失败。
以下是修改后的代码:
```
# 导入MySQL模块
import mysql.connector
# 创建MySQL连接
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 获取游标
mycursor = mydb.cursor()
def login():
# 获取输入框中的账号密码
username = username_entry.get()
password = password_entry.get()
# 查询MySQL表中是否存在该账号密码对应的记录
mycursor.execute("SELECT * FROM users WHERE username=%s AND password=%s", (username, password))
result = mycursor.fetchone()
if result:
print("登录成功!")
else:
print("登录失败!")
# 创建登录界面
login_frame = tk.Frame(root, bg='white')
login_frame.pack(pady=20)
# 添加账号、密码输入框和登录按钮
tk.Label(login_frame, text='账号:',bg='white').grid(row=0, column=0,pady=10)
username_entry = tk.Entry(login_frame,bd=2)
username_entry.grid(row=0, column=1,pady=10)
tk.Label(login_frame, text='密码:',bg='white').grid(row=1, column=0,pady=10)
password_entry = tk.Entry(login_frame, show='*',bd=2)
password_entry.grid(row=1, column=1,pady=10)
login_button = tk.Button(login_frame, text='登录', command=login)
login_button.grid(row=2, column=1, pady=10)
```
需要注意的是,上述代码中的MySQL连接信息和查询语句需要根据实际情况进行修改。
相关推荐
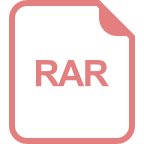
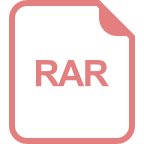
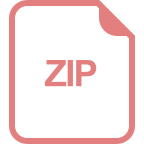












