C语言写程序给定一个文本字符串 s 和 m 个模板,每个模板用 {i}(0≤i≤9) 表示,对应一个字符串 ti ,请将 s 中的所有形如 {i} 的字符串替换为 ti ,如果 s 中包含多个 {i} ,则所有的 {i} 均需要被替换成 ti 。第一行包含一个字符串 s ,表示给定的文本字符串。s 的长度不超过 1000 ,保证 s 中非模板部分只出现英文字母、英文标点(不包括 { 和 } )及空格。 第二行包含一个整数 m(1≤m≤10) ,表示模板数量。 接下来 m 行,每行包含一个字符串 ti ,表示第 i 种模板。ti 的长度不超过 10 ,且只出现英文字母。测试用例为I have a {0}, I have an {1}. 2 pen apple结果应该为I have a pen, I have an apple.
时间: 2023-11-28 14:51:21 浏览: 117
以下是C语言的实现代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 1000
#define MAX_TEMPLATE 10
#define TEMPLATE_LEN 10
char s[MAX_LEN + 1];
char templates[MAX_TEMPLATE][TEMPLATE_LEN + 1];
char output[MAX_LEN * 10 + 1]; // 预留足够的空间,避免越界
int main() {
fgets(s, MAX_LEN + 1, stdin);
int s_len = strlen(s);
if (s[s_len - 1] == '\n') {
s[s_len - 1] = '\0'; // 去掉换行符
s_len--;
}
int m;
scanf("%d\n", &m);
for (int i = 0; i < m; i++) {
fgets(templates[i], TEMPLATE_LEN + 1, stdin);
int template_len = strlen(templates[i]);
if (templates[i][template_len - 1] == '\n') {
templates[i][template_len - 1] = '\0'; // 去掉换行符
template_len--;
}
}
int output_pos = 0;
for (int i = 0; i < s_len; i++) {
if (s[i] == '{') {
int j = i + 1;
while (j < s_len && s[j] != '}') {
j++;
}
if (j >= s_len) {
printf("Error: unmatched { found!\n");
return 1;
}
s[i] = '\0'; // 把 { 变成字符串结尾,截取出非模板部分
char* template_index_str = s + i + 1;
int template_index = atoi(template_index_str);
if (template_index < 0 || template_index >= m) {
printf("Error: invalid template index %d!\n", template_index);
return 1;
}
strcat(output, s + output_pos); // 把非模板部分拼接到输出字符串
strcat(output, templates[template_index]); // 把模板字符串拼接到输出字符串
output_pos = j + 1; // 跳过模板字符串
i = j; // 跳过模板字符串
}
}
strcat(output, s + output_pos); // 把剩余的非模板部分拼接到输出字符串
printf("%s\n", output);
return 0;
}
```
具体思路如下:
1. 读入字符串 `s` 和模板数量 `m`,以及 `m` 个模板字符串。
2. 从左到右遍历 `s`,如果遇到一个 `{`,则找到对应的 `}`,把中间的字符串解析成模板索引,然后把中间的非模板部分和模板字符串拼接到输出字符串中。
3. 最后把剩余的非模板部分拼接到输出字符串中。
4. 输出最终的字符串。
需要注意的细节:
1. 读入字符串时要注意去掉换行符。
2. 如果解析模板索引失败,需要返回错误。
3. 如果遇到未匹配的 `{` 或 `}`,需要返回错误。
4. 预留足够的输出字符串空间,避免越界。
阅读全文
相关推荐
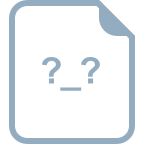




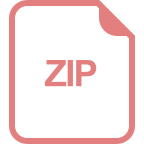
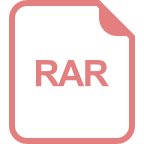
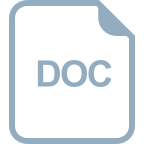
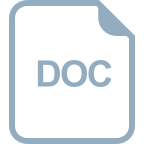
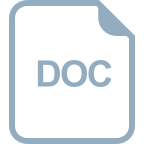
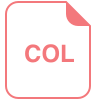
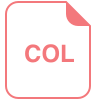
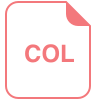
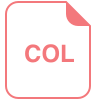
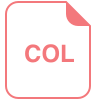