用Java swing gui 实现数独游戏的代码,要求1、要求产生指定形式的数独题目 2、在产生的数独题目上,程序产生数独的答案,将题目和答案保存在文件。 3、用户可按指定格式输入数独答案,程序可以检查答案是否正确,如果不正确,给出参考答案。
时间: 2023-12-03 10:44:45 浏览: 78
以下是一个基于Java Swing的数独游戏的简单示例代码。
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SudokuGame extends JFrame implements ActionListener {
private JTextField[][] puzzleFields;
private JButton checkButton;
private JButton newGameButton;
private JButton solveButton;
private int[][] solution;
private int[][] puzzle;
public SudokuGame() {
setTitle("数独游戏");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
// 创建数独面板
JPanel puzzlePanel = new JPanel(new GridLayout(9, 9));
contentPane.add(puzzlePanel, BorderLayout.CENTER);
puzzleFields = new JTextField[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
puzzleFields[i][j] = new JTextField();
puzzleFields[i][j].setHorizontalAlignment(JTextField.CENTER);
puzzlePanel.add(puzzleFields[i][j]);
}
}
// 创建按钮面板
JPanel buttonPanel = new JPanel(new FlowLayout());
contentPane.add(buttonPanel, BorderLayout.SOUTH);
checkButton = new JButton("检查答案");
checkButton.addActionListener(this);
buttonPanel.add(checkButton);
newGameButton = new JButton("新游戏");
newGameButton.addActionListener(this);
buttonPanel.add(newGameButton);
solveButton = new JButton("解题");
solveButton.addActionListener(this);
buttonPanel.add(solveButton);
// 初始化数独谜题和解决方案
puzzle = generatePuzzle();
solution = solvePuzzle(puzzle);
// 显示数独谜题
displayPuzzle(puzzle);
// 调整窗口大小并显示
pack();
setVisible(true);
}
// 生成新的数独谜题
private int[][] generatePuzzle() {
// TODO: 实现数独谜题生成算法
return new int[9][9];
}
// 显示数独谜题或解决方案
private void displayPuzzle(int[][] puzzle) {
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (puzzle[i][j] == 0) {
puzzleFields[i][j].setText("");
} else {
puzzleFields[i][j].setText("" + puzzle[i][j]);
puzzleFields[i][j].setEditable(false);
}
}
}
}
// 检查答案是否正确
private boolean checkAnswer() {
int[][] answer = new int[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
String text = puzzleFields[i][j].getText().trim();
if (text.length() > 0) {
try {
int value = Integer.parseInt(text);
if (value < 1 || value > 9) {
JOptionPane.showMessageDialog(this, "请输入1到9之间的数字!", "错误", JOptionPane.ERROR_MESSAGE);
return false;
}
answer[i][j] = value;
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "请输入1到9之间的数字!", "错误", JOptionPane.ERROR_MESSAGE);
return false;
}
} else {
answer[i][j] = 0;
}
}
}
return compareArrays(answer, solution);
}
// 解决数独谜题
private int[][] solvePuzzle(int[][] puzzle) {
// TODO: 实现数独谜题解决算法
return new int[9][9];
}
// 比较两个二维整型数组是否相等
private boolean compareArrays(int[][] array1, int[][] array2) {
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (array1[i][j] != array2[i][j]) {
return false;
}
}
}
return true;
}
// 处理按钮事件
public void actionPerformed(ActionEvent event) {
if (event.getSource() == checkButton) {
if (checkAnswer()) {
JOptionPane.showMessageDialog(this, "恭喜你,答案正确!", "提示", JOptionPane.INFORMATION_MESSAGE);
} else {
displayPuzzle(solution);
JOptionPane.showMessageDialog(this, "答案错误,参考答案已显示!", "提示", JOptionPane.WARNING_MESSAGE);
}
} else if (event.getSource() == newGameButton) {
puzzle = generatePuzzle();
solution = solvePuzzle(puzzle);
displayPuzzle(puzzle);
} else if (event.getSource() == solveButton) {
displayPuzzle(solution);
}
}
public static void main(String[] args) {
new SudokuGame();
}
}
```
该示例代码包含了以下功能:
1. 通过 `generatePuzzle` 方法生成数独谜题,并将其保存在 `puzzle` 数组中。
2. 通过 `solvePuzzle` 方法解决数独谜题,并将其保存在 `solution` 数组中。
3. 通过 `displayPuzzle` 方法显示数独谜题或解决方案。
4. 通过 `checkAnswer` 方法检查用户输入的答案是否正确,并在需要时显示参考答案。
5. 通过 `actionPerformed` 方法处理按钮事件,包括检查答案、生成新游戏和显示解决方案。
请注意,该示例代码中的 `generatePuzzle` 和 `solvePuzzle` 方法尚未实现。您需要根据自己的需求和算法实现这些方法。
阅读全文
相关推荐
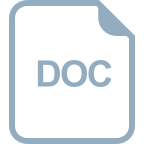
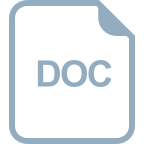
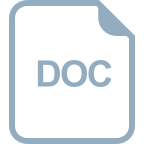


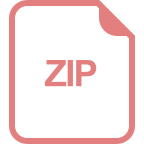
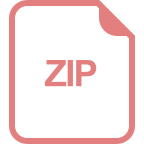
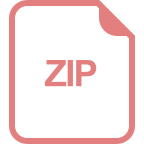
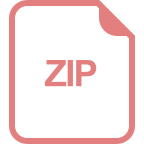
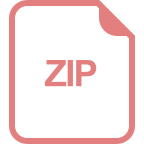
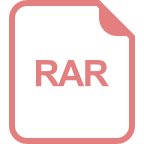
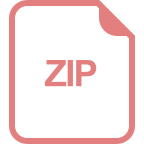
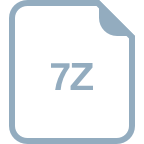
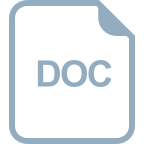
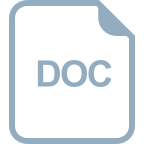
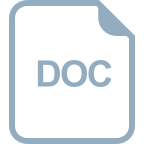
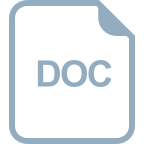
