写一个web3.js连接钱包的例子
时间: 2023-03-20 07:03:08 浏览: 129
当然,我可以回答你的问题。以下是一个基本的Web3.js连接钱包的示例:
```
// 引入Web3.js库
const Web3 = require('web3');
// 连接钱包
const connectWallet = async () => {
// 检查钱包是否已经安装
if (window.ethereum) {
try {
// 请求用户授权
await window.ethereum.enable();
// 实例化Web3对象
const web3 = new Web3(window.ethereum);
console.log('已成功连接钱包!');
return web3;
} catch (error) {
console.error('用户拒绝授权连接钱包!');
}
} else {
console.error('请安装MetaMask钱包!');
}
}
// 调用连接钱包函数
connectWallet();
```
这个例子中,我们使用Web3.js库来连接钱包,并通过MetaMask钱包进行用户授权。注意,这个示例仅仅是一个基本的示例,实际项目中需要更完善的错误处理、安全性考虑等。
相关问题
前端写web3.js调用钱包发送交易的例子
以下是使用Web3.js调用钱包发送交易的一个简单例子:
```
const Web3 = require('web3');
const Tx = require('ethereumjs-tx').Transaction;
const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR-PROJECT-ID'));
// 设置账户私钥和地址
const account = {
address: 'YOUR-ADDRESS',
privateKey: Buffer.from('YOUR-PRIVATE-KEY', 'hex')
};
// 设置转账信息
const toAddress = 'RECIPIENT-ADDRESS';
const value = web3.utils.toWei('1', 'ether');
// 获取账户的交易数
web3.eth.getTransactionCount(account.address, (err, txCount) => {
if (err) {
console.error(err);
return;
}
// 构造交易对象
const txObject = {
nonce: web3.utils.toHex(txCount),
to: toAddress,
value: web3.utils.toHex(value),
gasLimit: web3.utils.toHex(21000),
gasPrice: web3.utils.toHex(web3.utils.toWei('10', 'gwei'))
};
// 签名交易
const tx = new Tx(txObject, { 'chain': 'mainnet' });
tx.sign(account.privateKey);
// 序列化交易对象
const serializedTx = tx.serialize();
const rawTx = '0x' + serializedTx.toString('hex');
// 发送交易
web3.eth.sendSignedTransaction(rawTx, (err, txHash) => {
if (err) {
console.error(err);
return;
}
console.log(`Transaction hash: ${txHash}`);
});
});
```
在这个例子中,我们使用Web3.js连接到以太坊主网的Infura节点,设置了发送交易的账户地址和私钥,以及转账信息,构造交易对象,签名交易并序列化交易对象,最后发送交易。请注意,在实际使用中,您需要替换上面的变量和值为您自己的信息。
找一个web3.js的cdn
以下是web3.js的CDN链接:
https://cdn.jsdelivr.net/npm/web3@1.3.0/dist/web3.min.js
您可以将上述链接放入您的HTML文件中,如下所示:
```html
<script src="https://cdn.jsdelivr.net/npm/web3@1.3.0/dist/web3.min.js"></script>
```
这将在您的HTML文件中加载web3.js库。
相关推荐
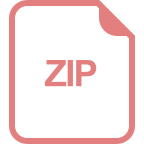
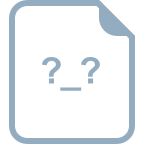












