import numpy as np import matplotlib.pyplot as plt from matplotlib.ticker import MaxNLocator # 创建画布和子图对象 fig, ax = plt.subplots(figsize=(9, 6), dpi=100) # 绘制折线图 ax.plot(x, y) # 绘制平均值线 #ax.axhline(y=-650, color='r', linestyle='--',label='流域整体物质平衡=-650mm w.e.') # 添加阴影带 start_year = 2006 end_year = 2016 mask = np.logical_and(years >= start_year, years <= end_year) years_to_plot = years[mask] ax.fill_between(years_to_plot, -680- 220, -680 + 220, alpha=0.2,color='yellow',label='Brun et al.2017') ax.axhline(-680, color='yellow', linestyle='--',xmin=0.65, xmax=0.89) start_year_2 = 2000 end_year_2 = 2014 mask_2 = np.logical_and(years >= start_year_2, years <= end_year_2) years_to_plot_2 = years[mask_2] ax.fill_between(years_to_plot_2, -790-110, -790+110, alpha=0.2, color='green',label='Wu et al.2018') ax.axhline(-790, color='green', linestyle='--',xmin=0.51, xmax=0.840) start_year_3 = 2000 end_year_3 = 2018 mask_3 = np.logical_and(years >= start_year_3, years <= end_year_3) years_to_plot_3 = years[mask_3] ax.fill_between(years_to_plot_3, -540-160, -540+160, alpha=0.2, color='blue',label='Shean et al.2020') ax.axhline(-540, color='blue', linestyle='--',xmin=0.51, xmax=0.93) start_year_4 = 2000 end_year_4 = 2019 mask_4 = np.logical_and(years >= start_year_4, years <= end_year_4) years_to_plot_4 = years[mask_4] ax.fill_between(years_to_plot_4, -580-220, -580+220, alpha=0.2, color='red',label='Hugonnet et al.2021') ax.axhline(-580, color='red', linestyle='--',xmin=0.51, xmax=0.957) # 设置 x 轴标签和标题 ax.set_xlabel('年份',fontproperties=font_prop,fontsize=14) ax.set_ylabel('物质平衡(mm w.e.)',fontproperties=font_prop,fontsize=14) ax.set_title('图8 帕隆藏布流域1980-2019物质平衡',fontproperties=font_prop,fontsize=14,y=-0.17) # 强制显示整数刻度 ax.xaxis.set_major_locator(MaxNLocator(integer=True)) # 添加网格 ax.grid(True, which='major', linestyle='--') # 将坐标轴的刻度字体大小设置为12 ax.tick_params(axis='both', which='major', labelsize=12) # 添加图例 ax.legend(fontsize=24,loc='lower left',prop=font_prop) # 设置图形的边距 plt.tight_layout() # 显示图形 plt.show()
时间: 2023-06-24 20:05:01 浏览: 217
该代码是用Python中的matplotlib库绘制折线图,并添加了四个阴影带,代表不同研究者的数据范围。其中,ax.plot(x, y)用于绘制折线图,ax.fill_between(years_to_plot, -680- 220, -680 + 220, alpha=0.2,color='yellow',label='Brun et al.2017')用于添加阴影带,ax.axhline(-680, color='yellow', linestyle='--',xmin=0.65, xmax=0.89)用于绘制平均值线。其他部分则是对图形进行美化,如添加图例、设置坐标轴标签和标题等。最后使用plt.show()将图形显示出来。
相关问题
优化import scipy.fftpack as fp from skimage.io import imread from skimage.color import rgb2gray, gray2rgb import matplotlib.pyplot as plt from skimage.draw import rectangle_perimeter import numpy as np import cv2 import numpy as np import matplotlib.pylab as plt from mpl_toolkits.mplot3d import Axes3D # noqa: F401 unused import from mpl_toolkits.axes_grid1 import make_axes_locatable from skimage.metrics import peak_signal_noise_ratio, structural_similarity from sklearn.metrics import mean_squared_error, mean_absolute_error from matplotlib.ticker import LinearLocator, FormatStrFormatter from scipy.fftpack import dct, idct import cv2 as cv def dct2(a): return dct(dct(a, axis=0, norm='ortho'), axis=1, norm='ortho') def idct2(a): return idct(idct(a, axis=0, norm='ortho'), axis=1, norm='ortho') im = rgb2gray(imread('2.jpg')) imF = dct2(im) im1 = idct2(imF) print(np.allclose(im, im1)) plt.figure(figsize=(10,5)) plt.gray() # 绘制第一个子图 plt.subplot(121), plt.imshow(im), plt.axis('off'), plt.title('original image', size=10) # 显示第一个子图 plt.show() # 绘制第二个子图 plt.subplot(122), plt.imshow(im1), plt.axis('off'), plt.title('reconstructed image (DCT+IDCT)', size=10) ,使能控制压缩率
为了控制压缩率,您可以使用DCT系数的阈值来减少它们的数量。具体来说,您可以将DCT系数的绝对值与一个阈值进行比较,并将小于该阈值的系数设置为零。这个阈值越高,压缩率就越高,但是图像质量也会受到影响。您可以使用以下代码来实现这一点:
```python
# 设置阈值
threshold = 0.1
# 对DCT系数进行阈值处理
imF_thresh = imF * (np.abs(imF) > (threshold*np.max(imF)))
# 对处理后的DCT系数进行IDCT反变换
im2 = idct2(imF_thresh)
# 显示处理前后的图像
fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(10, 5))
ax = axes.ravel()
ax[0].imshow(im, cmap=plt.cm.gray)
ax[0].set_title('Original image')
ax[1].imshow(im2, cmap=plt.cm.gray)
ax[1].set_title('Compressed image (DCT+IDCT)')
for a in ax:
a.axis('off')
plt.show()
```
在这个例子中,我们将阈值设置为0.1,即只保留最大DCT系数的10%。您可以根据需要调整这个值来控制压缩率和图像质量。
import matplotlib.ticker as mtick
`matplotlib.ticker`是Matplotlib库中用于绘制坐标轴刻度线和标签的工具包。`mtick`模块提供了一些常用的刻度线格式化器和定位器。比如可以使用`PercentFormatter`来将小数转换为百分数形式,也可以使用`MultipleLocator`来设置坐标轴刻度的间隔。
以下是一个简单例子,演示如何使用`mtick`模块来设置y轴刻度为百分数形式:
``` python
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
import numpy as np
x = np.linspace(0, 1, 11)
y = np.random.rand(11)
fig, ax = plt.subplots()
ax.plot(x, y)
# 设置y轴刻度为百分数形式
fmt = '%.0f%%' # 将小数转换为百分数,保留0位小数
yticks = mtick.FormatStrFormatter(fmt)
ax.yaxis.set_major_formatter(yticks)
plt.show()
```
运行以上代码,将会得到一张图像,y轴刻度显示为百分数形式。
阅读全文
相关推荐
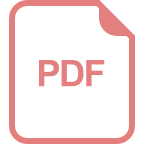
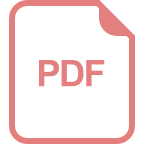
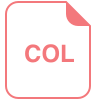
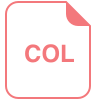
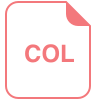
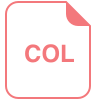
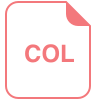
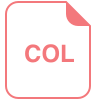







