用java帮我写一个上传图片
时间: 2023-09-21 08:06:28 浏览: 108
### 回答1:
好的,我可以帮您编写一个上传图片的Java程序。您需要使用Java的文件上传API,例如Apache Commons FileUpload或Servlet 3.文件上传API。您可以使用以下代码片段作为参考:
```
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
@WebServlet("/upload")
public class FileUploadServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String UPLOAD_DIRECTORY = "uploads";
private static final int MEMORY_THRESHOLD = 1024 * 1024 * 3; // 3MB
private static final int MAX_FILE_SIZE = 1024 * 1024 * 40; // 40MB
private static final int MAX_REQUEST_SIZE = 1024 * 1024 * 50; // 50MB
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// checks if the request actually contains upload file
if (!ServletFileUpload.isMultipartContent(request)) {
// if not, we stop here
response.getWriter().println("Error: Form must have enctype=multipart/form-data.");
response.flushBuffer();
return;
}
// configures upload settings
DiskFileItemFactory factory = new DiskFileItemFactory();
// sets memory threshold - beyond which files are stored in disk
factory.setSizeThreshold(MEMORY_THRESHOLD);
// sets temporary location to store files
factory.setRepository(new File(System.getProperty("java.io.tmpdir")));
ServletFileUpload upload = new ServletFileUpload(factory);
// sets maximum size of upload file
upload.setFileSizeMax(MAX_FILE_SIZE);
// sets maximum size of request (include file + form data)
upload.setSizeMax(MAX_REQUEST_SIZE);
// constructs the directory path to store upload file
String uploadPath = getServletContext().getRealPath("") + File.separator + UPLOAD_DIRECTORY;
// creates the directory if it does not exist
File uploadDir = new File(uploadPath);
if (!uploadDir.exists()) {
uploadDir.mkdir();
}
try {
// parses the request's content to extract file data
@SuppressWarnings("unchecked")
List<FileItem> formItems = upload.parseRequest(request);
if (formItems != null && formItems.size() > ) {
// iterates over form's fields
for (FileItem item : formItems) {
// processes only fields that are not form fields
if (!item.isFormField()) {
String fileName = new File(item.getName()).getName();
String filePath = uploadPath + File.separator + fileName;
File storeFile = new File(filePath);
// saves the file on disk
item.write(storeFile);
response.getWriter().println("Upload successful: " + fileName);
response.flushBuffer();
}
}
}
} catch (Exception ex) {
response.getWriter().println("Error: " + ex.getMessage());
response.flushBuffer();
}
}
}
```
请注意,您需要将上面的代码片段放在一个Servlet类中,并将其映射到您的Web应用程序中的URL。此外,您需要在Web应用程序的Web.xml文件中配置Servlet映射。
希望这可以帮助您完成上传图片的Java程序。
### 回答2:
当然可以使用Java编写一个上传图片的程序。以下是一个简单的示例:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class ImageUploader {
public static void uploadImage(String imageUrl, String destinationPath) throws IOException {
URL url = new URL(imageUrl);
try (InputStream in = url.openStream()) {
Path destination = Path.of(destinationPath);
Files.copy(in, destination, StandardCopyOption.REPLACE_EXISTING);
System.out.println("图片上传成功!");
} catch (IOException e) {
System.out.println("图片上传失败!");
}
}
public static void main(String[] args) {
String imageUrl = "http://example.com/image.jpg"; // 图片的URL地址
String destinationPath = "C:/path/to/destination/image.jpg"; // 图片保存的本地路径
try {
uploadImage(imageUrl, destinationPath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个程序使用`java.net.URL`来获取给定URL中的图片数据,并使用Java的`java.nio.file`包将图片保存到指定的本地路径。你可以根据自己的需求修改`imageUrl`和`destinationPath`的值。当运行程序时,它将尝试将图片从URL地址上传到本地路径,并在控制台上打印出上传成功或失败的消息。
请注意,这只是一个简单的示例,可能并不适用于所有情况。对于更复杂的需求,你可能需要进一步定制代码。
### 回答3:
在Java中,可以使用`javax.servlet`包提供的`MultipartConfigElement`和`Part`类来写一个上传图片的功能。
首先,需要在web.xml文件中配置一个servlet,以便处理上传图片的请求。在servlet配置中,需要设置servlet的映射路径和multipart配置。下面是一个示例的配置:
```xml
<servlet>
<servlet-name>UploadServlet</servlet-name>
<servlet-class>com.example.UploadServlet</servlet-class>
<multipart-config>
<location>/path/to/save/uploads</location> <!-- 上传图片保存的路径 -->
<max-file-size>1048576</max-file-size> <!-- 最大文件大小限制,这里设置为1MB -->
<max-request-size>2097152</max-request-size> <!-- 最大请求大小限制,这里设置为2MB -->
<file-size-threshold>0</file-size-threshold> <!-- 文件大小超过阈值时写入磁盘,默认为0表示全部写入磁盘 -->
</multipart-config>
</servlet>
<servlet-mapping>
<servlet-name>UploadServlet</servlet-name>
<url-pattern>/upload</url-pattern> <!-- servlet的访问路径 -->
</servlet-mapping>
```
接下来,创建一个`UploadServlet`类来处理上传图片的请求。在servlet的`doPost`方法中,可以通过`request.getPart`方法获取上传的图片数据,并将其保存到指定的路径上。下面是一个示例的UploadServlet类:
```java
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
import java.io.IOException;
@MultipartConfig
public class UploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String uploadPath = "/path/to/save/uploads"; // 设置上传图片保存的路径
Part filePart = request.getPart("file"); // 获取上传的图片数据
String fileName = filePart.getSubmittedFileName(); // 获取上传的图片文件名
// 保存上传的图片到指定路径
filePart.write(uploadPath + fileName);
response.getWriter().println("Upload successful!");
}
}
```
以上代码只是一个简单的示例来演示上传图片的功能,实际应用中可能需要进行更多的错误处理、文件名处理等。
使用时,可以通过发送一个POST请求到配置的servlet路径,将图片数据作为请求的`file`参数上传即可。
希望以上信息能帮到你,谢谢!
阅读全文
相关推荐


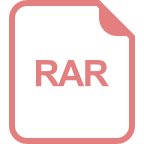










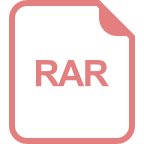
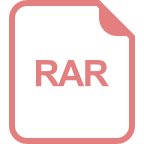