rt-thread studio实时读取天气并显示在串口屏上
时间: 2023-08-14 14:06:09 浏览: 70
以下是一个简单的示例代码,演示如何在 RT-Thread Studio 中实时读取天气信息并在串口屏上显示:
```c
#include <rtthread.h>
#include <rtdevice.h>
#include <finsh.h>
#include <stdlib.h>
#include <string.h>
#include <dfs_posix.h>
#include <dfs_file.h>
#include <dfs.h>
#include <shell.h>
#include <stdio.h>
#include <httpclient.h>
#include <json-c/json.h>
#include "bsp.h"
#include "serial_lcd.h"
#define WEATHER_API_KEY "your_api_key" // 替换成你的天气 API 密钥
#define WEATHER_API_URL "http://api.openweathermap.org/data/2.5/weather?q=shenzhen,cn&appid=" WEATHER_API_KEY
#define LCD_UART_NAME "uart2"
#define LCD_BAUDRATE 9600
static struct rt_serial_device lcd_serial;
static struct serial_lcd lcd;
static void lcd_init(void)
{
rt_err_t result;
struct serial_configure config = RT_SERIAL_CONFIG_DEFAULT;
config.baud_rate = LCD_BAUDRATE;
config.data_bits = DATA_BITS_8;
config.stop_bits = STOP_BITS_1;
config.parity = PARITY_NONE;
rt_memset(&lcd_serial, 0, sizeof(lcd_serial));
rt_memset(&lcd, 0, sizeof(lcd));
lcd_serial.ops = &uart_ops;
result = rt_serial_register(&lcd_serial, LCD_UART_NAME, &config, &lcd);
if (result != RT_EOK) {
rt_kprintf("Failed to register serial device for LCD, error: %d\n", result);
return;
}
serial_lcd_init(&lcd);
}
static void get_weather_data(void)
{
char url[256];
char response[1024];
struct httpclient_data httpclient;
json_object *root, *weather, *main, *temp, *humidity;
int temperature, weather_id, humidity_value;
char buffer[64];
// 拼接 API 请求地址
rt_snprintf(url, sizeof(url), WEATHER_API_URL);
// 初始化 HTTP 客户端
httpclient_data_init(&httpclient);
httpclient_set_server_url(&httpclient, url);
// 发送 HTTP 请求
if (httpclient_get(&httpclient) != HTTPCLIENT_OK) {
rt_kprintf("Failed to get weather data, error: %s\n", httpclient.errmsg);
goto exit;
}
// 解析返回的 JSON 数据
root = json_tokener_parse(response);
if (json_object_get_type(root) != json_type_object) {
rt_kprintf("Failed to parse weather data\n");
goto exit;
}
// 提取温度、湿度、天气状况等信息
if (!json_object_object_get_ex(root, "main", &main) ||
!json_object_object_get_ex(main, "temp", &temp) ||
!json_object_object_get_ex(main, "humidity", &humidity) ||
!json_object_object_get_ex(root, "weather", &weather)) {
rt_kprintf("Failed to extract weather data\n");
goto exit;
}
temperature = (int)json_object_get_double(temp) - 273; // 温度单位为开尔文,转换为摄氏度
humidity_value = (int)json_object_get_double(humidity);
weather_id = (int)json_object_get_int(json_object_array_get_idx(weather, 0));
// 在串口屏上显示天气信息
rt_snprintf(buffer, sizeof(buffer), "Temperature: %dC\nHumidity: %d%%\n", temperature, humidity_value);
serial_lcd_clear(&lcd);
serial_lcd_puts(&lcd, buffer);
exit:
httpclient_data_deinit(&httpclient);
json_object_put(root);
}
static void get_weather_thread_entry(void* parameter)
{
while (1) {
get_weather_data();
rt_thread_delay(30000); // 每 30 秒更新一次天气数据
}
}
int get_weather(void)
{
rt_thread_t tid;
rt_err_t result;
lcd_init();
tid = rt_thread_create("weather", get_weather_thread_entry, RT_NULL, 2048, 25, 5);
if (tid != RT_NULL) {
rt_thread_startup(tid);
return 0;
} else {
return -1;
}
}
// 在 RT-Thread Studio 命令行中输入 get_weather 命令即可启动天气获取线程
MSH_CMD_EXPORT(get_weather, get weather data);
```
在这个示例代码中,我们首先在串口屏上初始化 LCD 并通过 HTTP 请求访问天气 API 获取天气数据,然后解析返回的 JSON 数据,提取需要的温度、湿度等信息。最后使用串口屏显示天气信息,并使用一个线程每 30 秒更新一次天气数据。需要注意的是,串口屏的显示需要使用串口通信协议,本示例使用的是9600波特率的串口通信。
相关推荐
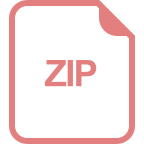
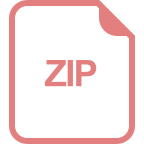
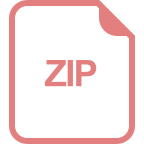














