STM32串口接收数据怎么使用printf查看接收到的字符串不使用中断
时间: 2024-03-16 18:48:02 浏览: 29
除了使用中断方式接收串口数据外,还可以使用轮询方式接收串口数据并使用printf函数打印接收到的字符串。以下是一个示例代码:
```
#include "stdio.h"
#include "string.h"
#include "stm32f4xx.h"
#define RX_BUFFER_SIZE 512
uint8_t rx_buffer[RX_BUFFER_SIZE]; // 定义接收缓存区
uint16_t rx_counter = 0; // 定义接收计数器
int main(void)
{
// 初始化串口
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_USART2);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE);
while (1)
{
if (USART_GetFlagStatus(USART2, USART_FLAG_RXNE) == SET)
{
uint8_t data = USART_ReceiveData(USART2);
rx_buffer[rx_counter++] = data;
if (data == '\n' || rx_counter >= RX_BUFFER_SIZE)
{
rx_buffer[rx_counter] = '\0'; // 在字符串末尾添加'\0'
printf("Received string: %s\n", rx_buffer);
rx_counter = 0; // 接收计数器清零
}
}
// 主循环中进行其他操作
}
}
```
在主循环中使用轮询方式检测串口接收标志位,当接收到字符'\n'时或者接收计数器达到缓存区大小时,将接收缓存区中的字符串打印到串口或者调试助手上,然后将接收计数器清零。
需要注意的是,在使用printf函数进行调试时,需要在工程设置中开启printf重定向功能。具体操作方法可以参考相关资料或者开发板的用户手册。
相关推荐
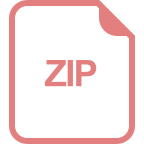
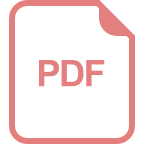
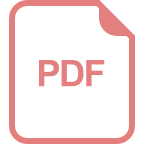














