用gstreamer C语言实现音视频同步的代码
时间: 2024-01-21 13:16:40 浏览: 173
以下是一个简单的使用 gstreamer C 语言实现音视频同步的代码示例:
```
#include <gst/gst.h>
static gboolean bus_call(GstBus *bus, GstMessage *msg, gpointer data) {
GMainLoop *loop = (GMainLoop *)data;
switch (GST_MESSAGE_TYPE(msg)) {
case GST_MESSAGE_EOS:
g_print("End of stream\n");
g_main_loop_quit(loop);
break;
case GST_MESSAGE_ERROR: {
gchar *debug;
GError *error;
gst_message_parse_error(msg, &error, &debug);
g_free(debug);
g_printerr("Error: %s\n", error->message);
g_error_free(error);
g_main_loop_quit(loop);
break;
}
default:
break;
}
return TRUE;
}
int main(int argc, char *argv[]) {
GMainLoop *loop;
GstElement *pipeline, *src, *sink, *audio_sink, *video_sink, *convert;
GstBus *bus;
guint bus_watch_id;
GstPad *video_pad, *audio_pad;
/* Initialize GStreamer */
gst_init(&argc, &argv);
/* Create the elements */
src = gst_element_factory_make("filesrc", "source");
convert = gst_element_factory_make("decodebin", "convert");
audio_sink = gst_element_factory_make("autoaudiosink", "audio_sink");
video_sink = gst_element_factory_make("autovideosink", "video_sink");
/* Create the empty pipeline */
pipeline = gst_pipeline_new("test-pipeline");
if (!pipeline || !src || !convert || !audio_sink || !video_sink) {
g_printerr("Not all elements could be created.\n");
return -1;
}
/* Build the pipeline */
gst_bin_add_many(GST_BIN(pipeline), src, convert, audio_sink, video_sink, NULL);
if (gst_element_link(src, convert) != TRUE ||
gst_element_link(convert, audio_sink) != TRUE ||
gst_element_link(convert, video_sink) != TRUE) {
g_printerr("Elements could not be linked.\n");
gst_object_unref(pipeline);
return -1;
}
/* Set the URI to play */
g_object_set(src, "location", argv[1], NULL);
/* Connect to the bus */
loop = g_main_loop_new(NULL, FALSE);
bus = gst_pipeline_get_bus(GST_PIPELINE(pipeline));
bus_watch_id = gst_bus_add_watch(bus, bus_call, loop);
gst_object_unref(bus);
/* Start playing */
gst_element_set_state(pipeline, GST_STATE_PLAYING);
/* Wait for the playback to finish */
g_main_loop_run(loop);
/* Clean up */
gst_element_set_state(pipeline, GST_STATE_NULL);
gst_object_unref(GST_OBJECT(pipeline));
g_source_remove(bus_watch_id);
g_main_loop_unref(loop);
return 0;
}
```
这个示例代码使用了 GStreamer 库来实现音视频播放,首先需要创建各个元素(例如`filesrc`、`decodebin`、`autoaudiosink`、`autovideosink`),然后将它们添加到管道中,并且使用`gst_element_link`函数将它们连接起来。接着,设置要播放的音视频文件的 URI,并将管道设置为播放状态。最后,使用`g_main_loop_run`函数等待播放结束。在播放过程中,使用`bus_call`函数来处理各种 GStreamer 消息,例如错误消息和结束消息。
在音视频同步方面,需要使用`gst_pad_set_offset`函数来设置音频和视频之间的时间偏移值,以确保它们能够同步播放。在管道中连接`decodebin`元素后,可以使用`gst_element_get_static_pad`函数获取音频和视频源的输入端口,并使用`gst_pad_set_offset`函数设置它们之间的时间偏移值。
由于每个音视频文件的编码和格式都不同,因此需要根据实际情况进行修改和调整。
阅读全文
相关推荐
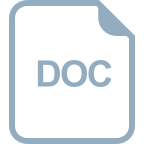
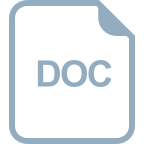
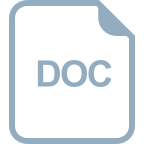
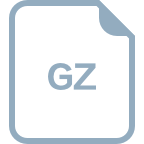
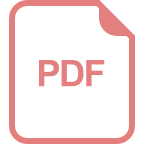
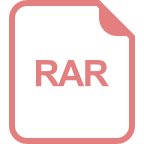
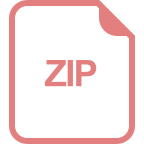
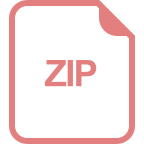
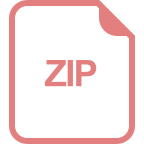
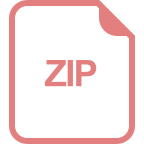
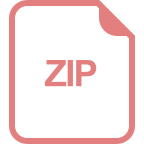
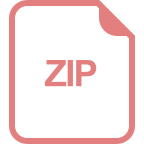
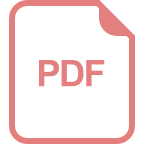
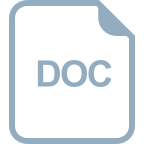
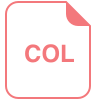
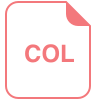
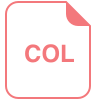
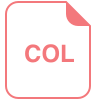
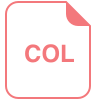