用gstreamer实现音视频同步的代码
时间: 2024-01-21 17:16:38 浏览: 113

gstreamer源代码

以下是一个简单的用 GStreamer 实现音视频同步的示例代码:
```python
import gi
gi.require_version('Gst', '1.0')
from gi.repository import Gst, GObject
GObject.threads_init()
Gst.init(None)
player = Gst.ElementFactory.make("playbin", "player")
player.set_property("uri", "file:///path/to/media/file")
audio_sink = Gst.ElementFactory.make("autoaudiosink", "audio_sink")
video_sink = Gst.ElementFactory.make("autovideosink", "video_sink")
player.set_property("audio-sink", audio_sink)
player.set_property("video-sink", video_sink)
bus = player.get_bus()
def on_message(bus, message):
t = message.type
if t == Gst.MessageType.EOS:
player.set_state(Gst.State.NULL)
loop.quit()
elif t == Gst.MessageType.ERROR:
err, debug = message.parse_error()
print ("Error: %s" % err, debug)
player.set_state(Gst.State.NULL)
loop.quit()
elif t == Gst.MessageType.STATE_CHANGED:
if message.src == player:
old_state, new_state, pending_state = message.parse_state_changed()
if new_state == Gst.State.PLAYING:
# Get the current time when playing starts
query = Gst.Query.new_seeking(Gst.Format.TIME)
if player.query(query):
_, start, _ = query.parse_seeking()
global start_time
start_time = start
elif t == Gst.MessageType.QOS:
# Get the running time of the pipeline
query = Gst.Query.new_position(Gst.Format.TIME)
if player.query(query):
_, running_time = query.parse_position()
running_time += start_time
# Get the running time of the last buffer
struct = message.get_structure()
_, running_time_buffer, _ = struct.get("running-time")
running_time_buffer += start_time
# Calculate the difference between the two running times
diff = running_time - running_time_buffer
# If the difference is too big, seek to the correct position
if abs(diff) > Gst.SECOND / 10:
player.seek_simple(Gst.Format.TIME, Gst.SeekFlags.FLUSH | Gst.SeekFlags.KEY_UNIT, running_time)
bus.add_signal_watch()
bus.connect("message", on_message)
# Start playing
player.set_state(Gst.State.PLAYING)
# Start the main loop
loop = GObject.MainLoop()
loop.run()
```
此示例中使用了 `autoaudiosink` 和 `autovideosink` 作为音视频的输出,你还可以将其替换为其他的 sink。在收到 QOS 消息时,获取管道的当前时间以及最后一个缓冲区的运行时间,并在两个运行时间之间计算差异,如果差异太大,则使用 `seek_simple()` 函数跳转到正确的位置,以保持音视频同步。
阅读全文
相关推荐

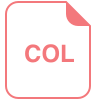
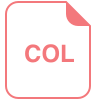
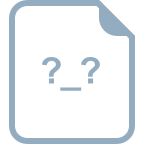
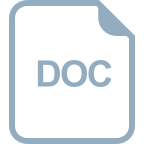
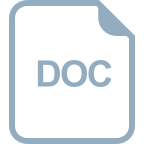
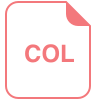
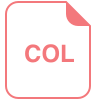
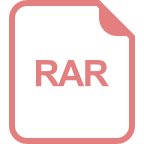
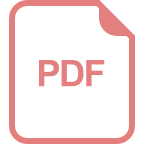
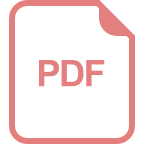
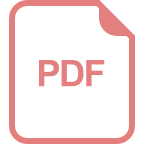
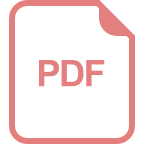
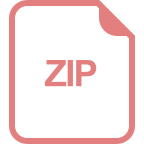
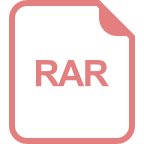
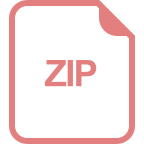
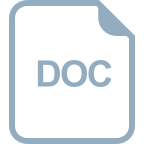