将pycharm输出窗口的内容捕获并实时更新发送到ui窗口中的QTextEdit上
时间: 2024-02-24 10:57:20 浏览: 25
可以使用Python中的`subprocess`模块来捕获PyCharm输出窗口的内容,并使用PyQt中的`QTextEdit`来实时更新UI窗口。
以下是一个简单的示例代码:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit
from PyQt5.QtCore import QThread, pyqtSignal
import subprocess
class ConsoleThread(QThread):
output = pyqtSignal(str)
def __init__(self, parent=None):
super().__init__(parent)
def run(self):
process = subprocess.Popen(['python', '-u', 'your_script.py'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
while True:
output = process.stdout.readline().decode().strip()
if output:
self.output.emit(output)
class MainWindow(QMainWindow):
def __init__(self, parent=None):
super().__init__(parent)
self.text_edit = QTextEdit()
self.setCentralWidget(self.text_edit)
self.console_thread = ConsoleThread()
self.console_thread.output.connect(self.update_text_edit)
self.console_thread.start()
def update_text_edit(self, text):
self.text_edit.append(text)
if __name__ == '__main__':
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
```
在上述代码中,`ConsoleThread`继承自`QThread`,用于在后台运行PyCharm输出窗口的内容。`run`方法使用`subprocess`模块启动一个子进程并捕获其输出。如果输出不为空,则通过`output`信号将其发送给UI线程。
在`MainWindow`中,我们创建了一个`QTextEdit`用于显示PyCharm输出窗口的内容。我们还创建了一个`ConsoleThread`并连接了其`output`信号到`update_text_edit`槽函数。在`update_text_edit`中,我们使用`append`方法将新的文本追加到`QTextEdit`中。
最后,我们创建了一个`QApplication`并显示`MainWindow`。当应用程序运行时,`ConsoleThread`将在后台捕获并发送PyCharm输出窗口的内容,而`MainWindow`将实时更新`QTextEdit`中的文本。
相关推荐
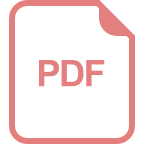
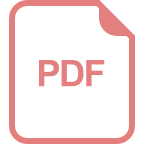














