unity按钮围绕视频大范围旋转
时间: 2023-07-26 10:45:57 浏览: 47
要实现Unity按钮围绕视频大范围旋转,可以使用以下步骤:
1. 在Unity中创建一个空对象,并将视频对象和按钮对象作为其子对象。
2. 将空对象的Transform组件的Position属性设置为(0,0,0),Rotation属性设置为(0,0,0)。
3. 创建一个C#脚本,并将其绑定到空对象上。
4. 在脚本中定义一个公共变量,用于控制旋转速度。
5. 在Update()函数中,使用Transform.RotateAround()方法使空对象围绕Y轴旋转,从而实现按钮和视频的大范围旋转。
以下是示例代码:
```csharp
using UnityEngine;
public class RotateAround : MonoBehaviour
{
public Transform target;
public float speed = 10f;
void Update()
{
transform.RotateAround(target.position, Vector3.up, speed * Time.deltaTime);
}
}
```
在这个示例中,target变量是视频对象的Transform组件。你可以通过拖拽视频对象到空对象的Inspector面板中来设置这个变量。speed变量控制旋转速度,可以通过Inspector面板或在代码中进行设置。
相关推荐
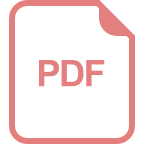
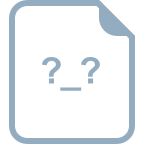
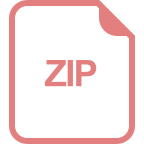
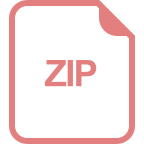
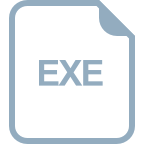
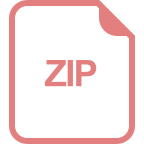