golang如何优雅的实现线程池
时间: 2023-07-17 17:55:43 浏览: 262
在Go语言中,可以通过使用goroutine和channel来实现线程池。下面是一个优雅实现线程池的示例代码:
```go
package main
import (
"fmt"
"sync"
)
type ThreadPool struct {
workerCount int
taskQueue chan func()
wg sync.WaitGroup
}
func NewThreadPool(workerCount int) *ThreadPool {
tp := &ThreadPool{
workerCount: workerCount,
taskQueue: make(chan func()),
}
tp.start()
return tp
}
func (tp *ThreadPool) start() {
for i := 0; i < tp.workerCount; i++ {
go func() {
for task := range tp.taskQueue {
task()
tp.wg.Done()
}
}()
}
}
func (tp *ThreadPool) AddTask(task func()) {
tp.wg.Add(1)
tp.taskQueue <- task
}
func (tp *ThreadPool) Wait() {
tp.wg.Wait()
}
func main() {
// 创建线程池,指定线程数量为5
pool := NewThreadPool(5)
// 添加任务到线程池
for i := 0; i < 10; i++ {
taskID := i
pool.AddTask(func() {
fmt.Printf("Task %d is running\n", taskID)
})
}
// 等待所有任务完成
pool.Wait()
}
```
在上面的代码中,首先定义了一个`ThreadPool`结构体,包含了`workerCount`表示线程数量和`taskQueue`表示任务队列。`NewThreadPool`函数用于创建一个新的线程池,同时启动对应数量的goroutine来处理任务队列中的任务。
线程池通过`AddTask`方法添加任务,任务以函数的形式传入。`Wait`方法用于等待所有任务完成,使用了`sync.WaitGroup`来实现。
在`main`函数中,创建了一个线程池并添加了10个任务。每个任务都会打印自己的ID。
通过这种方式,可以灵活控制并发任务的数量,并且可以重复使用线程池来处理多个任务。
阅读全文
相关推荐
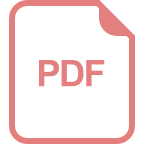
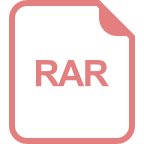
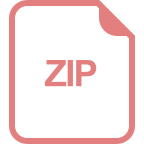
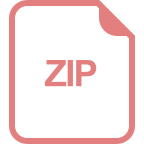
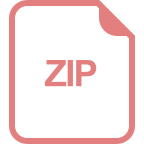
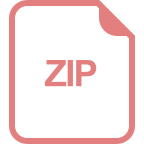
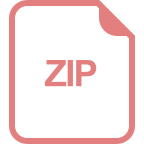
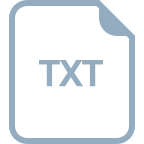
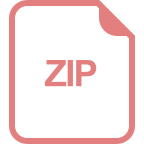
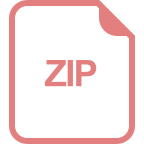
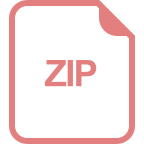
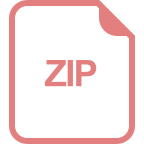
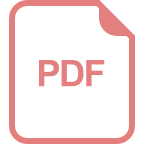
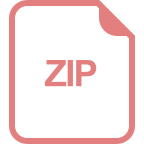
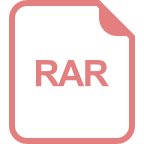
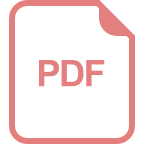
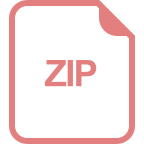
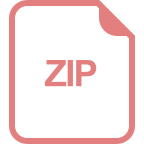