module jfq_612(out,data,load,reset,clk); output[3:0] out; input[3:0] data; input load,clk,reset; reg[3:0] out; always @(posedge clk,negedge reset) begin if(! reset)out=4'h00; else if(load) out=data; else out=out+1; end endmodule 修改为同步置数,异步清零的4位加法计数器
时间: 2024-03-26 11:40:46 浏览: 47
下面是修改后的代码:
```
module sync_reset_counter(out, clk, reset);
output reg [3:0] out;
input clk, reset;
always @(posedge clk) begin
if (reset)
out <= 4'h0; // 异步清零
else
out <= out + 1; // 同步置数
end
endmodule
```
这个模块只有一个输出端口 `out` 和两个输入端口 `clk` 和 `reset`。每当时钟上升沿到来时,如果 `reset` 信号为 `1`,则输出端口 `out` 会被异步清零;否则,`out` 会被同步置数(加一)。
相关问题
module jfq_8(a,b,cin,cout,dout); input [7:0] a,b; input cin; output [7:0] dout; output cout; wire [8:0] DATA; assign DATA=a+b+cin; assign cout=DATA[8]; assign dout=DATA[7:0]; endmodule 写出这段代码的测试文件
下面是针对 jfq_8 模块的测试文件示例:
```
module jfq_8_test;
// Declare inputs and outputs
reg [7:0] a;
reg [7:0] b;
reg cin;
wire [7:0] dout;
wire cout;
// Instantiate the module to be tested
jfq_8 dut(
.a(a),
.b(b),
.cin(cin),
.cout(cout),
.dout(dout)
);
// Assign inputs
initial begin
a = 8'h01;
b = 8'h02;
cin = 1'b0;
end
// Test case 1
initial begin
#10;
$display("Test case 1:");
$display("a = %h, b = %h, cin = %b", a, b, cin);
#5;
$display("dout = %h, cout = %b", dout, cout);
#5;
if (dout === 8'h03 && cout === 1'b0) begin
$display("Test case 1 passed!");
end else begin
$display("Test case 1 failed!");
end
#10;
end
// Test case 2
initial begin
a = 8'hff;
b = 8'h01;
cin = 1'b1;
#10;
$display("Test case 2:");
$display("a = %h, b = %h, cin = %b", a, b, cin);
#5;
$display("dout = %h, cout = %b", dout, cout);
#5;
if (dout === 8'h01 && cout === 1'b1) begin
$display("Test case 2 passed!");
end else begin
$display("Test case 2 failed!");
end
#10;
end
endmodule
```
该测试文件包含两个测试用例,分别测试了 jfq_8 模块对于不同输入的输出是否符合预期。在实际使用中,可以根据需要编写更多的测试用例。
module jfq_605(S,C_out,A,B,C_in); input A,B,C_in ; output S,C_out ; reg S,C_out ; always @(A or B or C_in) begin {C_out,S} = A +B +C_in ; end endmodule 写出这段代码的测试文件
下面是这段代码的测试文件,可以用于仿真或者实际的硬件验证:
```
`timescale 1ns / 1ps
module test_jfq_605;
// Inputs
reg A;
reg B;
reg C_in;
// Outputs
wire S;
wire C_out;
// Instantiate the Unit Under Test (UUT)
jfq_605 uut (
.A(A),
.B(B),
.C_in(C_in),
.S(S),
.C_out(C_out)
);
initial begin
// Initialize Inputs
A = 0;
B = 0;
C_in = 0;
// Wait 100 ns for global reset to finish
#100;
// Add stimulus here
// Test case 1: A=0, B=0, C_in=0
A = 0;
B = 0;
C_in = 0;
#10;
if (S !== 0 || C_out !== 0) $error("Test case 1 failed");
// Test case 2: A=0, B=0, C_in=1
A = 0;
B = 0;
C_in = 1;
#10;
if (S !== 1 || C_out !== 0) $error("Test case 2 failed");
// Test case 3: A=0, B=1, C_in=0
A = 0;
B = 1;
C_in = 0;
#10;
if (S !== 1 || C_out !== 0) $error("Test case 3 failed");
// Test case 4: A=0, B=1, C_in=1
A = 0;
B = 1;
C_in = 1;
#10;
if (S !== 0 || C_out !== 1) $error("Test case 4 failed");
// Test case 5: A=1, B=0, C_in=0
A = 1;
B = 0;
C_in = 0;
#10;
if (S !== 1 || C_out !== 0) $error("Test case 5 failed");
// Test case 6: A=1, B=0, C_in=1
A = 1;
B = 0;
C_in = 1;
#10;
if (S !== 0 || C_out !== 1) $error("Test case 6 failed");
// Test case 7: A=1, B=1, C_in=0
A = 1;
B = 1;
C_in = 0;
#10;
if (S !== 0 || C_out !== 1) $error("Test case 7 failed");
// Test case 8: A=1, B=1, C_in=1
A = 1;
B = 1;
C_in = 1;
#10;
if (S !== 1 || C_out !== 1) $error("Test case 8 failed");
$display("All test cases passed");
// End testbench
$finish;
end
endmodule
```
这个测试文件有8个测试用例,分别对应了所有可能的输入情况。每个测试用例会在A、B、C_in输入信号上给定不同的值,并且等待一段时间,以便UUT产生输出。之后会检查S和C_out输出信号的值是否符合预期,如果不符合则会报错。最后,如果所有的测试用例都通过了,就会显示"All test cases passed"。
阅读全文
相关推荐
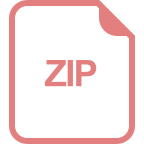
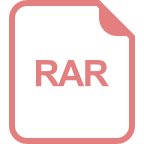
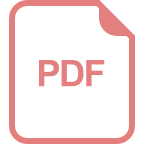
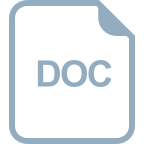
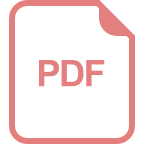
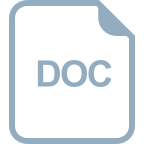
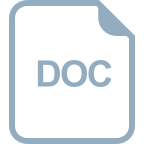
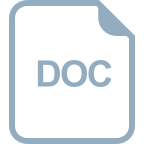


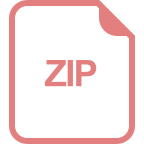
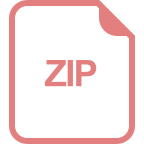