用matlab编写filter函数并与其他函数比较结果简单示例
时间: 2024-09-14 18:16:25 浏览: 38
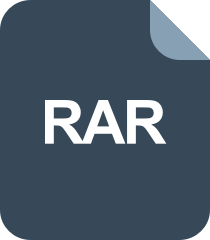
filter.rar_Chebyshev_butter_cheby2_filter 函数_matlab filter函数
在MATLAB中,`filter` 函数是一个内置工具,用于数字信号处理,它能够对连续时间信号进行滤波操作,例如低通、高通、带通或带阻滤波等。以下是创建一个简单的低通滤波器并比较其结果的一个示例:
```Matlab
% 定义信号源(假设我们有一个正弦波)
t = 0:0.01:5; % 时间轴
f_s = 100; % 采样频率
x = sin(2*pi*5*t); % 5Hz正弦波
% 设计低通滤波器,比如一个Butterworth滤波器
cutoff_freq = 10; % 截止频率
fs = f_s; % 采样频率
[b, a] = butter(4, cutoff_freq/(fs/2), 'low'); % 4阶Butterworth滤波器设计
% 使用filter函数进行滤波
y_filtered = filter(b, a, x);
% 比较原信号和滤波后的信号
figure;
plot(t, x, 'b', t, y_filtered, 'r');
title('Original Signal vs Filtered Signal');
xlabel('Time (s)');
ylabel('Amplitude');
legend('Original', 'Filtered');
% 计算过滤效果,如均方误差
mse = mean((x - y_filtered).^2);
fprintf('Mean Square Error: %f\n', mse);
阅读全文
相关推荐
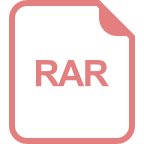
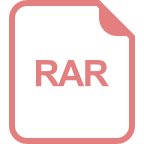

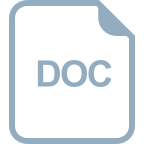
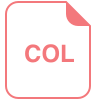











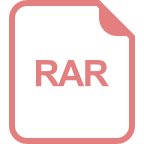
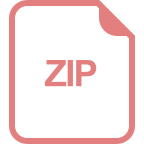
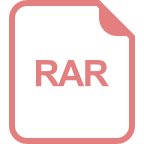