springboot + kotlin 更新接口详细例子
时间: 2023-11-11 11:54:08 浏览: 47
以下是使用 Spring Boot 和 Kotlin 更新 API 的详细示例:
1. 创建一个新的 Spring Boot 项目并添加必要的依赖项,如下所示:
```gradle
dependencies {
implementation("org.springframework.boot:spring-boot-starter-web")
implementation("org.jetbrains.kotlin:kotlin-stdlib-jdk8")
implementation("org.jetbrains.kotlin:kotlin-reflect")
}
```
2. 创建一个名为 `User` 的数据类,用于表示要更新的用户:
```kotlin
data class User(
val id: Long,
val name: String,
val email: String
)
```
3. 创建一个名为 `UserController` 的控制器类,用于处理用户更新请求:
```kotlin
@RestController
@RequestMapping("/api/users")
class UserController(private val userRepository: UserRepository) {
@PutMapping("/{id}")
fun updateUser(@PathVariable id: Long, @RequestBody user: User): User {
val existingUser = userRepository.findById(id)
.orElseThrow { EntityNotFoundException("User with id $id not found") }
existingUser.name = user.name
existingUser.email = user.email
return userRepository.save(existingUser)
}
}
```
4. 创建一个名为 `UserRepository` 的存储库接口,用于访问用户数据:
```kotlin
@Repository
interface UserRepository : JpaRepository<UserEntity, Long>
```
5. 创建一个名为 `UserEntity` 的实体类,用于映射到数据库中的用户表:
```kotlin
@Entity
@Table(name = "users")
data class UserEntity(
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
val id: Long = 0,
@Column(nullable = false)
var name: String,
@Column(nullable = false, unique = true)
var email: String
)
```
6. 运行应用程序并使用 cURL 或其他 HTTP 客户端发出更新用户的请求:
```bash
curl -X PUT -H "Content-Type: application/json" -d '{"name": "John Doe", "email": "john.doe@example.com"}' http://localhost:8080/api/users/1
```
这将更新 ID 为 1 的用户的名称和电子邮件,并返回更新后的用户对象。
相关推荐
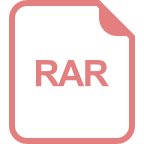














