qt接收数据实时显示并绘制成折线图在widget中
时间: 2023-12-30 09:06:18 浏览: 211
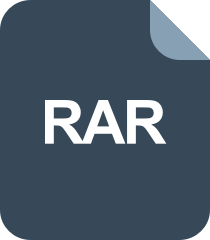
tchart和chart折线图实时显示

要在Qt的QWidget中实时显示并绘制数据折线图,可以参考以下步骤:
1. 在QWidget中添加一个QCustomPlot控件用于绘制折线图。
2. 在QWidget的构造函数中,创建一个Qtimer对象,用于定时更新数据。
3. 在Qtimer的槽函数中,读取数据并更新折线图。
4. 在QCustomPlot控件的初始化函数中,设置x和y轴范围、坐标轴标签等属性。
5. 在更新折线图的槽函数中,先将新的数据添加到QVector容器中,再将容器中的数据绘制成折线图。
下面是一个简单的代码示例,仅供参考:
```
// QWidget类
class MyWidget : public QWidget
{
Q_OBJECT
public:
explicit MyWidget(QWidget *parent = 0);
~MyWidget();
private slots:
void updateData(); // 定时更新数据的槽函数
void plotGraph(); // 绘制折线图的槽函数
private:
QCustomPlot *m_customPlot; // 绘制折线图的QCustomPlot控件
QTimer *m_timer; // 定时器对象
QVector<double> m_xData, m_yData; // 数据容器
};
// 构造函数
MyWidget::MyWidget(QWidget *parent) :
QWidget(parent)
{
// 创建QCustomPlot控件
m_customPlot = new QCustomPlot(this);
// 创建定时器对象
m_timer = new QTimer(this);
connect(m_timer, SIGNAL(timeout()), this, SLOT(updateData()));
m_timer->start(100); // 每100ms更新一次数据
// 初始化QCustomPlot控件
m_customPlot->addGraph();
m_customPlot->graph(0)->setPen(QPen(Qt::blue));
m_customPlot->xAxis->setLabel("Time");
m_customPlot->yAxis->setLabel("Value");
m_customPlot->xAxis->setRange(0, 10);
m_customPlot->yAxis->setRange(0, 100);
// 设置布局
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(m_customPlot);
setLayout(layout);
}
// 析构函数
MyWidget::~MyWidget()
{
}
// 定时更新数据的槽函数
void MyWidget::updateData()
{
// 读取数据...
// 处理数据...
plotGraph(); // 更新折线图
}
// 绘制折线图的槽函数
void MyWidget::plotGraph()
{
double x = QDateTime::currentDateTime().toMSecsSinceEpoch()/1000.0; // 获取当前时间
double y = 50; // 假设读取到的数据为50
m_xData.append(x);
m_yData.append(y);
// 绘制折线图
m_customPlot->graph(0)->setData(m_xData, m_yData);
m_customPlot->replot();
}
```
阅读全文
相关推荐
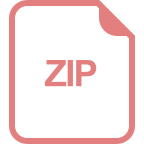
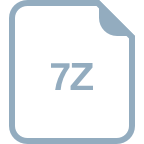











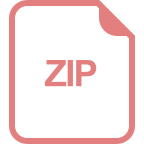
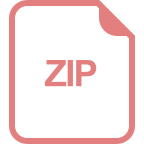
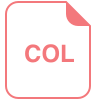

